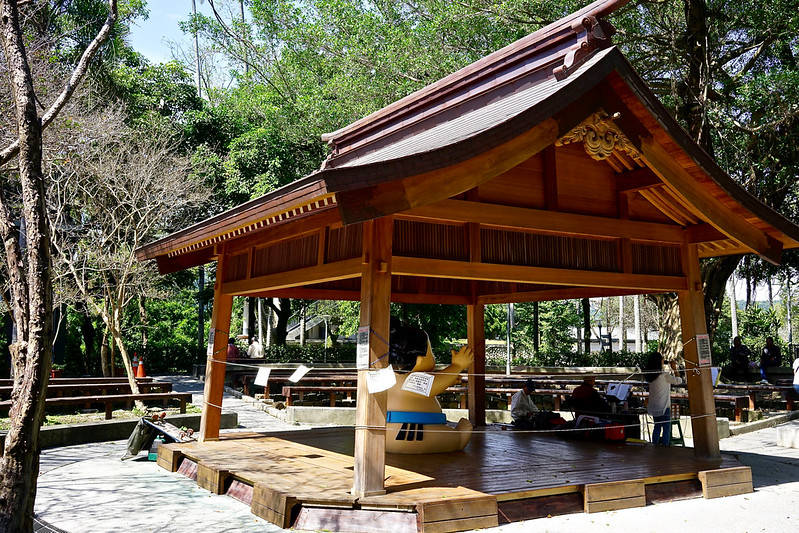
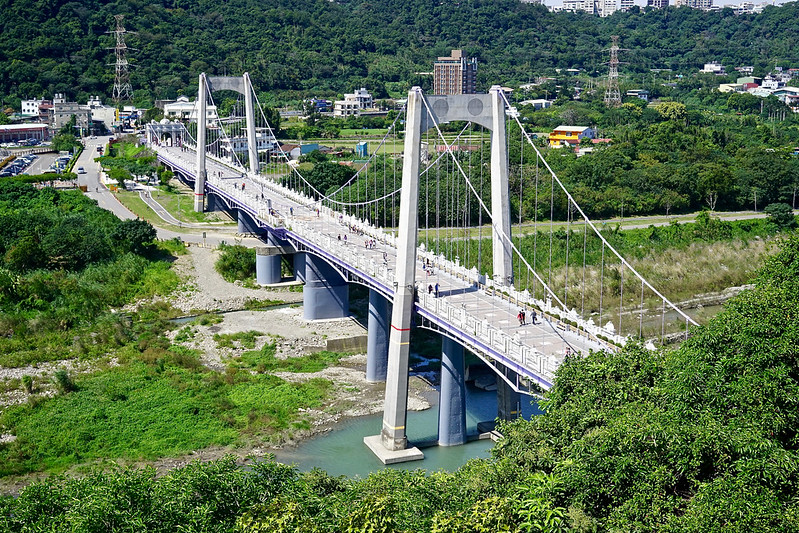

木藝生態博物館壹號館


武德殿

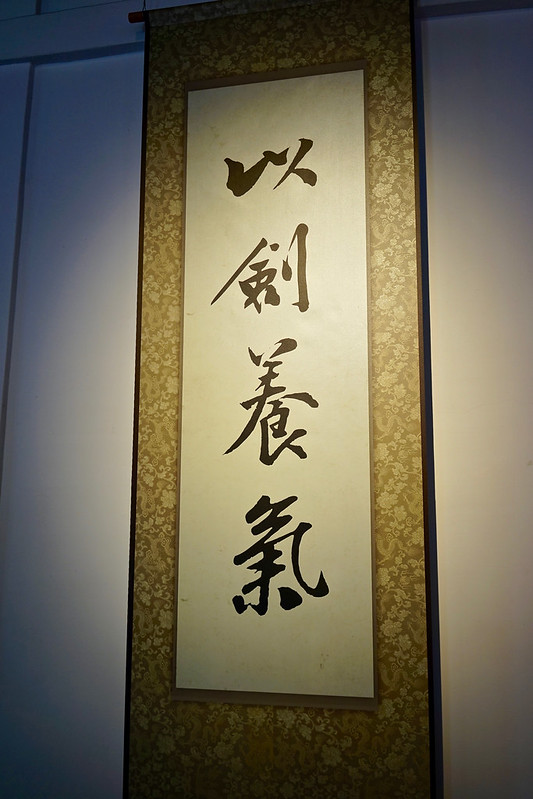

match (doc:Doctor)-[r1:PRESCRIBES]->(med:Medicine) where r1.date >= "20180401" and r1.date <= "20180415" with doc.name as doctor, med.name as medicine, count(med.name) as prescription_frequency return doctor, medicine, prescription_frequency order by prescription_frequency desc
match (doc:Doctor)-[r1:PRESCRIBES]->(med:Medicine) where r1.date >= "20180401" and r1.date <= "20180415" with doc.name as doctor, med.name as medicine, count(med.name) as prescription_frequency where prescription_frequency >= 3 return doctor, medicine, prescription_frequency order by prescription_frequency desc
package springboot.rest.controller; import java.util.ArrayList; import java.util.List; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import springboot.rest.vo.Book; @RestController @RequestMapping("/api/books") public class BookController { private static List<Book> books = new ArrayList<Book>(); static { Book book1 = new Book("1501108085", "Peaks and Valleys", "Spencer Johnson M.D."); Book book2 = new Book("0062102419", "How Will You Measure Your Life?", "Clayton M. Christensen"); Book book3 = new Book("111908833X", "Bogle On Mutual Funds", "John C. Bogle"); books.add(book1); books.add(book2); books.add(book3); } @GetMapping public String getBooks() throws JsonProcessingException { String booksJSON = ""; try { ObjectMapper objectMapper = new ObjectMapper(); booksJSON = objectMapper.writeValueAsString(books); } catch (JsonProcessingException e) { throw e; } return booksJSON; } @PostMapping(value = "/add") public String addBook(@RequestBody Book book) throws JsonProcessingException { books.add(book); String booksJSON = ""; try { ObjectMapper objectMapper = new ObjectMapper(); booksJSON = objectMapper.writeValueAsString(books); } catch (JsonProcessingException e) { throw e; } return booksJSON; } @PutMapping(value = "/update") public String updateBook(@RequestBody Book book) throws JsonProcessingException { books.stream().forEach(b -> { if (b.getName().equals(book.getName())) { b.setIsbn(book.getIsbn()); } }); String booksJSON = ""; try { ObjectMapper objectMapper = new ObjectMapper(); booksJSON = objectMapper.writeValueAsString(books); } catch (JsonProcessingException e) { throw e; } return booksJSON; } @DeleteMapping(value = "/delete/{isbn}") public String deleteBook(@PathVariable String isbn) throws JsonProcessingException { books.removeIf(b -> b.getIsbn().equals(isbn)); String booksJSON = ""; try { ObjectMapper objectMapper = new ObjectMapper(); booksJSON = objectMapper.writeValueAsString(books); } catch (JsonProcessingException e) { throw e; } return booksJSON; } }
package springboot.rest.vo; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import lombok.ToString; @Data @AllArgsConstructor @NoArgsConstructor @ToString public class Book { private String isbn; private String name; private String author; }
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>springboot</groupId> <artifactId>rest</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>rest</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <jackson.version>2.9.5</jackson.version> <springboot.version>2.0.0.RELEASE</springboot.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>${springboot.version}</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <version>${springboot.version}</version> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.16.18</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>${jackson.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>${jackson.version}</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
package test.albert.rest.client; import java.io.IOException; import java.io.UnsupportedEncodingException; import java.util.Date; import org.apache.commons.httpclient.HttpClient; import org.apache.commons.httpclient.HttpStatus; import org.apache.commons.httpclient.methods.GetMethod; import org.apache.commons.httpclient.methods.PostMethod; import org.apache.commons.httpclient.methods.StringRequestEntity; import com.fasterxml.jackson.annotation.JsonFormat; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import lombok.ToString; import lombok.extern.slf4j.Slf4j; @Slf4j public class RestClient { private final String GET_URL = "http://localhost:8080/api/greeting/get/sayHi?name=Albert"; private final String POST_URL = "http://localhost:8080/api/greeting/post/sayHi"; public static void main(String[] args) throws IOException { RestClient client = new RestClient(); client.doGetRequest(); client.doPostRequest(); } public void doGetRequest() throws IOException { HttpClient client = new HttpClient(); GetMethod getMethod = new GetMethod(GET_URL); try { int statusCode = client.executeMethod(getMethod); if (statusCode != HttpStatus.SC_OK) { String error = "do Get Request failed: " + getMethod.getStatusLine(); throw new RuntimeException(error); } String responseBodyStr = getMethod.getResponseBodyAsString(); log.debug("[doGetRequest] responseBodyStr = " + responseBodyStr); } catch (IOException e) { throw e; } finally { getMethod.releaseConnection(); } } public void doPostRequest() throws JsonProcessingException, UnsupportedEncodingException { String greetingJson = createJSON(); StringRequestEntity requestEntity = new StringRequestEntity(greetingJson, "application/json", "UTF-8"); HttpClient client = new HttpClient(); PostMethod postMethod = new PostMethod(POST_URL); postMethod.setRequestEntity(requestEntity); try { int statusCode = client.executeMethod(postMethod); if (statusCode != HttpStatus.SC_OK) { String error = "do Post Request failed: " + postMethod.getStatusLine(); throw new RuntimeException(error); } String responseStr = postMethod.getResponseBodyAsString(); log.debug("[doPostRequest] responseStr = " + responseStr); } catch (IOException e) { e.printStackTrace(); } finally { postMethod.releaseConnection(); } } private String createJSON() throws JsonProcessingException { Greeting greeting = new Greeting("Hello, Mandy", null); return new ObjectMapper().writeValueAsString(greeting); } @AllArgsConstructor @NoArgsConstructor @Data @ToString private static class Greeting { private String content; @JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd HH:mm:ss.SSS", timezone = "GMT+8") private Date datetime; } }
<dependency> <groupId>commons-httpclient</groupId> <artifactId>commons-httpclient</artifactId> <version>3.1</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.9.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.9.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.9.3</version> </dependency>
package springboot.rest.controller; import java.util.Date; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import springboot.rest.vo.Greeting; @RestController @RequestMapping("/api/greeting") public class GreetingController { @GetMapping("/get/sayHi") public String greeting(@RequestParam(value = "name") String name) throws JsonProcessingException { Greeting greeting = new Greeting("Hello, " + name, new Date()); String greetingJson = ""; try { ObjectMapper objectMapper = new ObjectMapper(); greetingJson = objectMapper.writeValueAsString(greeting); } catch (JsonProcessingException e) { throw e; } return greetingJson; } @PostMapping(value = "/post/sayHi") public String greeting(@RequestBody Greeting greeting) throws JsonProcessingException { Greeting greetingVo = new Greeting(greeting.getContent(), new Date()); String greetingJson = ""; try { ObjectMapper objectMapper = new ObjectMapper(); greetingJson = objectMapper.writeValueAsString(greetingVo); } catch (JsonProcessingException e) { throw e; } return greetingJson; } }
package springboot.rest.vo; import java.util.Date; import com.fasterxml.jackson.annotation.JsonFormat; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import lombok.ToString; @AllArgsConstructor @NoArgsConstructor @Data @ToString public class Greeting { private String content; @JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd HH:mm:ss.SSS", timezone = "GMT+8") private Date datetime; }
package springboot.rest; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class App { public static void main(String[] args) { SpringApplication.run(App.class, args); } }
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>springboot</groupId> <artifactId>rest</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>rest</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <jackson.version>2.9.5</jackson.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>2.0.0.RELEASE</version> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.16.18</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>${jackson.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>${jackson.version}</version> </dependency> </dependencies> </project>