Assume I have a simple requirement, I hope it will print current timestamp each 3 seconds in background.
Steps are as bellows:
1. Define background scripts in manifest.json
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | { "manifest_version": 2, "name": "Build Chrome Extension With AngularJS", "description": "利用 AngularJS 建置 Chrome Extension ", "version": "1.0", "permissions": [ "activeTab", "storage"], "browser_action": { "default_icon": "img/icon.png", "default_popup": "todo.html", "default_title": "Build Chrome Extension With AngularJS" }, "commands": { "_execute_browser_action": { "suggested_key": { "default": "Alt+Shift+D" } } }, "background": { "scripts": ["js/background.js"] }, "options_page": "options.html" } |
2. Create background.js
1 2 3 4 5 6 7 8 9 10 11 12 13 | var myVar = setInterval(myTimer, 3000); function myTimer() { var now = new Date(); var year = now.getFullYear(); var month = now.getMonth() + 1; var date = now.getDate(); var hour = now.getHours(); var minutes = now.getMinutes(); var seconds = now.getSeconds(); var time = year + '/' + month + '/' + date + ' ' + hour + ':' + minutes + ':' + seconds; console.log(time); } |
3. Reload extensions and open background page to test
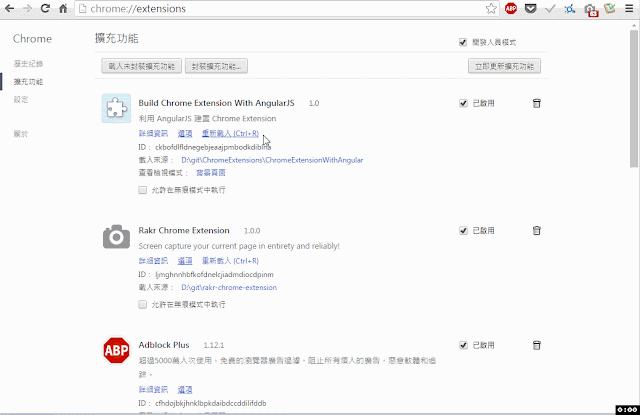