億載金城
台南市移民署
四草紅樹林綠色隧道
台南林百貨
善化胡厝寮
鹿耳門天后宮
台南火車站
Total Pageviews
2014/10/31
2014/10/29
How to read Excel file by Apache POI
Requirement
Assume we have an excel file, we would like to read the data which in sheet2.
We only need the amount in Column E and column I, and the value of Column A show be 'XXX年'
Here has sample code (focus on Line 17~33)
Here is the log which print in console
Reference
[1] http://viralpatel.net/blogs/java-read-write-excel-file-apache-poi/
Assume we have an excel file, we would like to read the data which in sheet2.
We only need the amount in Column E and column I, and the value of Column A show be 'XXX年'
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | public class POITest { private final static Logger LOG = LoggerFactory.getLogger(POITest.class); /** * The main method. * * @param args * the arguments * @throws IOException * Signals that an I/O exception has occurred. */ public static void main(String[] args) throws IOException { String testFile = "D:\\work\\ifms\\TestFiles\\4102294114W75Y7OW0.xls"; Integer sheetNum = 1; FileInputStream file = null; try { file = new FileInputStream(new File(testFile)); // Get the workbook instance for XLS file HSSFWorkbook workbook = new HSSFWorkbook(file); // Get the specific sheet from the workbook HSSFSheet sheet = workbook.getSheetAt(sheetNum); // Get iterator to all the rows in current sheet Iterator rowIterator = sheet.iterator(); List list = new ArrayList<>(); while (rowIterator.hasNext()) { Row row = rowIterator.next(); if (StringUtils.indexOf(row.getCell(0).toString(), "年") > 0) {//condition Test test = new POITest().new Test(); test.setYear(row.getCell(0).toString());//column A test.setGdp(new BigDecimal(row.getCell(4).getNumericCellValue()));//column E test.setGnp(new BigDecimal(row.getCell(8).getNumericCellValue()));//column I list.add(test); } } for (Test vo : list) { LOG.debug("vo=" + vo.toString()); } } catch (FileNotFoundException e) { throw new RuntimeException(e); } catch (IOException e) { throw new RuntimeException(e); } finally { file.close(); } } private class Test { private String year; private BigDecimal gdp; private BigDecimal gnp; public String getYear() { return year; } public void setYear(String year) { this.year = year; } public BigDecimal getGdp() { return gdp; } public void setGdp(BigDecimal gdp) { this.gdp = gdp; } public BigDecimal getGnp() { return gnp; } public void setGnp(BigDecimal gnp) { this.gnp = gnp; } public String toString() { return ToStringBuilder.reflectionToString(this); } } } |
Here is the log which print in console
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@2d7349d7[year=80年,gdp=4958220,gnp=5093770] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@6bf4d990[year=81年,gdp=5534544,gnp=5655306] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@17f7b44f[year=82年,gdp=6110101,gnp=6224145] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@75ebad4[year=83年,gdp=6685505,gnp=6793022] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@5c3bb813[year=84年,gdp=7277545,gnp=7388464] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@54b216b3[year=85年,gdp=7906075,gnp=8015577] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@77f06d35[year=86年,gdp=8574784,gnp=8664395] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@4991f017[year=87年,gdp=9204174,gnp=9272725] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@c299bbd[year=88年,gdp=9649049,gnp=9739567] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@7faf9b87[year=89年,gdp=10187394,gnp=10326952] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@620bfd8e[year=90年,gdp=9930387,gnp=10122411] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@133a7ec[year=91年,gdp=10411639,gnp=10654141] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@66557791[year=92年,gdp=10696257,gnp=11025130] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@751d0513[year=93年,gdp=11365292,gnp=11737391] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@44385e76[year=94年,gdp=11740279,gnp=12031145] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@50c1b7f7[year=95年,gdp=12243471,gnp=12555170] [main] DEBUG g.n.e.w.c.Ets402rReportController - vo=gov.nta.ets.web.controller.Ets402rReportController$Test@5e14e28c[year=96年,gdp=12910511,gnp=13243277] |
Reference
[1] http://viralpatel.net/blogs/java-read-write-excel-file-apache-poi/
2014/10/27
How to use Google Guava to do Collection Filter
Requirement
We have a collection data (List of value object), we would like to find the objects which fyr is 101.
Here has the collection data:
1: dtoList=[
2: gov.nta.fms.dto.FmsSumRateDto@1a395e5a[fyr=101,accmon=01,alc=168407392348.53,alcavg=0.0809],
3: gov.nta.fms.dto.FmsSumRateDto@2c617429[fyr=101,accmon=02,alc=164834284030.53,alcavg=0.0792],
4: gov.nta.fms.dto.FmsSumRateDto@32114682[fyr=101,accmon=03,alc=180965071697.53,alcavg=0.0869],
5: gov.nta.fms.dto.FmsSumRateDto@c2c1a7c[fyr=101,accmon=04,alc=165659472439.53,alcavg=0.0796],
6: gov.nta.fms.dto.FmsSumRateDto@237ec922[fyr=101,accmon=05,alc=180372232532.53,alcavg=0.0866],
7: gov.nta.fms.dto.FmsSumRateDto@58c6e962[fyr=101,accmon=06,alc=176357775471.53,alcavg=0.0847],
8: gov.nta.fms.dto.FmsSumRateDto@6b044e76[fyr=101,accmon=07,alc=187368376522.53,alcavg=0.09],
9: gov.nta.fms.dto.FmsSumRateDto@67bb5bdd[fyr=101,accmon=08,alc=181824695849.53,alcavg=0.0873],
10: gov.nta.fms.dto.FmsSumRateDto@3d8c3f5f[fyr=101,accmon=09,alc=170308922011.45,alcavg=0.0818],
11: gov.nta.fms.dto.FmsSumRateDto@4294958a[fyr=101,accmon=10,alc=168308032423.95,alcavg=0.0808],
12: gov.nta.fms.dto.FmsSumRateDto@28e5b92c[fyr=101,accmon=11,alc=179491195734.95,alcavg=0.0862],
13: gov.nta.fms.dto.FmsSumRateDto@1e00cae[fyr=101,accmon=12,alc=157844063362.95,alcavg=0.0758],
14: gov.nta.fms.dto.FmsSumRateDto@1c607478[fyr=102,accmon=01,alc=170969213258.95,alcavg=0.0806],
15: gov.nta.fms.dto.FmsSumRateDto@7e80d6[fyr=102,accmon=02,alc=159840653037.95,alcavg=0.0753],
16: gov.nta.fms.dto.FmsSumRateDto@713c61da[fyr=102,accmon=03,alc=169579370948.95,alcavg=0.0799],
17: gov.nta.fms.dto.FmsSumRateDto@333c694a[fyr=102,accmon=04,alc=169624940547.95,alcavg=0.0799],
18: gov.nta.fms.dto.FmsSumRateDto@4324938d[fyr=102,accmon=05,alc=173064277154.95,alcavg=0.0816],
19: gov.nta.fms.dto.FmsSumRateDto@1653306b[fyr=102,accmon=06,alc=169616844409.95,alcavg=0.0799],
20: gov.nta.fms.dto.FmsSumRateDto@2f8fcc40[fyr=102,accmon=07,alc=175060305269.95,alcavg=0.0825],
21: gov.nta.fms.dto.FmsSumRateDto@9aa9625[fyr=102,accmon=08,alc=193638114494.95,alcavg=0.0913],
22: gov.nta.fms.dto.FmsSumRateDto@1e675c18[fyr=102,accmon=09,alc=198567712906.95,alcavg=0.0936],
23: gov.nta.fms.dto.FmsSumRateDto@13cf3b40[fyr=102,accmon=10,alc=181427982270.95,alcavg=0.0855],
24: gov.nta.fms.dto.FmsSumRateDto@a3af0e9[fyr=102,accmon=11,alc=187353709301.95,alcavg=0.0883],
25: gov.nta.fms.dto.FmsSumRateDto@239e563e[fyr=102,accmon=12,alc=172980508042.95,alcavg=0.0815]
26: ]
How to Use Google Guava API
1. Create Predicate instance to determines a true or false value for a given input. (Line1~Line7)
2. Utilize Collection2.filter to return the elements of unfiltered that satisfy a predicate.(Line8~line9)
Here has the code snippet:
1: Predicate predicate = new Predicate() {
2: @Override
3: public boolean apply(FmsSumRateDto input) {
4: //Determines true or false value for a given input.
5: return "101".equqls(input.getFyr());
6: }
7: };
8: //Returns the elements of unfiltered that satisfy a predicate.
9: Iterator result = Collections2.filter(dtoList, predicate).iterator();
10: while(result.hasNext()) {
11: log.debug("result="+result.next().toString());
12: }
Here is the result which statisfy the predicate:
1: result=gov.nta.fms.dto.FmsSumRateDto@1a395e5a[fyr=101,accmon=01,alc=168407392348.53,alcavg=0.0809]
2: result=gov.nta.fms.dto.FmsSumRateDto@2c617429[fyr=101,accmon=02,alc=164834284030.53,alcavg=0.0792]
3: result=gov.nta.fms.dto.FmsSumRateDto@32114682[fyr=101,accmon=03,alc=180965071697.53,alcavg=0.0869]
4: result=gov.nta.fms.dto.FmsSumRateDto@c2c1a7c[fyr=101,accmon=04,alc=165659472439.53,alcavg=0.0796]
5: result=gov.nta.fms.dto.FmsSumRateDto@237ec922[fyr=101,accmon=05,alc=180372232532.53,alcavg=0.0866]
6: result=gov.nta.fms.dto.FmsSumRateDto@58c6e962[fyr=101,accmon=06,alc=176357775471.53,alcavg=0.0847]
7: result=gov.nta.fms.dto.FmsSumRateDto@6b044e76[fyr=101,accmon=07,alc=187368376522.53,alcavg=0.09]
8: result=gov.nta.fms.dto.FmsSumRateDto@67bb5bdd[fyr=101,accmon=08,alc=181824695849.53,alcavg=0.0873]
9: result=gov.nta.fms.dto.FmsSumRateDto@3d8c3f5f[fyr=101,accmon=09,alc=170308922011.45,alcavg=0.0818]
10: result=gov.nta.fms.dto.FmsSumRateDto@4294958a[fyr=101,accmon=10,alc=168308032423.95,alcavg=0.0808]
11: result=gov.nta.fms.dto.FmsSumRateDto@28e5b92c[fyr=101,accmon=11,alc=179491195734.95,alcavg=0.0862]
12: result=gov.nta.fms.dto.FmsSumRateDto@1e00cae[fyr=101,accmon=12,alc=157844063362.95,alcavg=0.0758]
Reference
[3] http://docs.guava-libraries.googlecode.com/git/javadoc/com/google/common/collect/Collections2.html
Labels:
Java
2014/10/23
要去哪裡查詢零股交易的相關資訊
有時候股票除了配現金以外,還會給零股給投資大眾,為了讓買賣比較容易(因為零股只能利用盤後交易),故通常會利用盤後交易來買零股湊成一張
我們如果要去查詢零股最佳一檔買、賣價格的話,可以到基本市況報導網站( http://mis.twse.com.tw/stock/oddTrade.jsp ) 查詢
有關零股更多資訊可以參考 http://www.masterlink.com.tw/service/qanda/Q&A-6.htm
Labels:
Investment
2014/10/21
How to copy text from Notepad++ to Microsoft Word and keep its color to distinguish keywords
Notepad++ can distinguish between different languages source code can be written in. For example, a language could distinguish certain keywords that have to be differently interpreted, and as such it can be useful to distinguish these keywords using another color or font.
For example.
The language is Java.
The language is SQL.
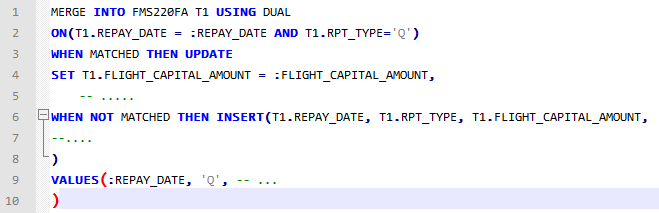
Scenario
If we would like to copy SQL statement from Notepad++ to Microsoft Word in my system design document, but it seems cannot keep its color to distinguish keywords.
For example.
Resolution
Step1. Select text you would like to copy.
Step2. Plugins --> NppExport --> Copy RTF to clipboard
Step3. Paste text to Microsoft word
Labels:
Tools
2014/10/16
How to display Tradition Chinese Character in Git Bash
Problem
Why I cannot display Tradition Chinese Character in Git Bash correctly?
Solution
You need to edit three files under git\etc1. edit gitconfig file and append configuration as following:
1: [gui]
2: encoding = utf-8
3: #log编码
4: [i18n]
5: commitencoding = utf-8
6: #支持中文路径
7: [svn]
8: pathnameencoding = utf-8
2. edit git-completion.bash file and append configuration as following:
1: #正常顯示中文
2: alias ls='ls --show-control-chars --color=auto'
3. edit inputrc file and append configuration as following:
1: #bash中可以正常输入中文
2: set output-meta on
3: set convert-meta off
Check Result
2014/10/15
JRebel - Reload any changes without restart server
As a developer, we do not want to waste time to restart server again and again to see the implace of code changes.
You can try JRebel to save your time.
If you are using Eclipse, here has good step-by-step installation and configuration guide: http://manuals.zeroturnaround.com/jrebel/ide/eclipse.html
For more information, please check http://zeroturnaround.com/software/jrebel/learn/
Demo
Here has code snippet, and it will print debug message as entering this method
And the console will print "test" debugging message as entering this method:
In order to test JRebel, I just modified the debug message to "JRebel test" and submit query without restart application server
It's because of JRebel reload any changes classes automatically.
When you change any class or resource in your IDE, the change will reflect in you application immediately, skipping the build and redeploy phases.
You can try JRebel to save your time.
If you are using Eclipse, here has good step-by-step installation and configuration guide: http://manuals.zeroturnaround.com/jrebel/ide/eclipse.html
For more information, please check http://zeroturnaround.com/software/jrebel/learn/
Demo
Here has code snippet, and it will print debug message as entering this method
1 2 3 4 5 6 7 8 9 10 11 12 | @RequestMapping(value = "/query/tab1", method = RequestMethod.POST, consumes = MediaType.APPLICATION_JSON_VALUE, produces = MediaType.APPLICATION_JSON_VALUE) public @ResponseBody List findTab1(@RequestBody Fms420rFormBean formBean, Alerter alerter) { log.debug("test"); List dataList = service.queryTab1(formBean.getYear(), formBean.getMonth()); if (CollectionUtils.isEmpty(dataList)) { alerter.info(Messages.warning_notFound()); } else { alerter.info(Messages.success_find()); } return dataList; } |
1: 19:15:45,493 INFO [stdout] [20123] DEBUG gov.nta.fms.web.rest.Fms420rResource - test
In order to test JRebel, I just modified the debug message to "JRebel test" and submit query without restart application server
1
2
3
4
5
6
7
8
9
10
11
12
@RequestMapping(value = "/query/tab1", method = RequestMethod.POST, consumes = MediaType.APPLICATION_JSON_VALUE, produces = MediaType.APPLICATION_JSON_VALUE)
public @ResponseBody
List findTab1(@RequestBody Fms420rFormBean formBean, Alerter alerter) {
log.debug("JRebel test");
List dataList = service.queryTab1(formBean.getYear(), formBean.getMonth());
if (CollectionUtils.isEmpty(dataList)) {
alerter.info(Messages.warning_notFound());
} else {
alerter.info(Messages.success_find());
}
return dataList;
}
See....I don't redeploy and restart application server, but the console print the up-to-date debug message. 1: 19:16:19,742 INFO [stdout] [20123] DEBUG gov.nta.fms.web.rest.Fms420rResource - JRebel test
It's because of JRebel reload any changes classes automatically.
When you change any class or resource in your IDE, the change will reflect in you application immediately, skipping the build and redeploy phases.
2014/10/14
How to find out the PID (process ID) of processes in Windows
Problem
If I would like to find out the PID of javaw.java proecess, how do I do?
Windows task manager seems does not have PID information.
Solution 1
View-->Selected Columns-->Checked PID-->OK
Then we can find out the PID information.
If I would like to find out the PID of javaw.java proecess, how do I do?
Windows task manager seems does not have PID information.
Solution 1
View-->Selected Columns-->Checked PID-->OK
Then we can find out the PID information.
Solution 2
Open command prompt, and execute tasklist /fi "Imagename eq JAVAW.exe"
Then we can get the PID information
映像名稱 PID 工作階段名稱 工作階段 # RAM使用量
========================= ======== ================ =========== ============
javaw.exe 10368 Console 1 1,620,884 K
Reference
Labels:
MicrosoftWindows
2014/10/01
[閱讀筆記] 拆開獲利的糖衣
- 債券價格的變動與利率負相關,利率上升,債券價格會下跌;利率下降,債券價格會上漲
- 短期投資來說,股票的波動大於債券。但是,若是拉長到30年,股票的波動只有債券的一半,但報酬好很多
- 波動是投資主要風險,但不是唯一風險。對許多長期投資者來說,如果採用波動不夠大的投資組合,這樣的投資成本長期看來會造成更大的壞處
- 多頭市場是在悲觀中生成,在懷疑中成長,在樂觀中成熟,在亢奮中消逝
- 股市是經濟的領先指標,投資人不會等經濟資料顯示經濟復甦才開始投資,他們會提前哄抬股價
- 如果你的投資期間很長,長期來說,由於貨幣升貶本質是零和、不規則的循環,貨幣對全球投資組合的影響會互相抵消,接近于零
- 貨幣的波動很大,長期來說,投資人很難掌握短期的市場時機,靠交易外匯獲利
- 如果每個人都在關注某一件事,你知道你可以放心忽略那件事,往別的方向看,關注大家沒注意的事,以及可能對未來市場走向有很大影響的事
- 衡量市場氛圍往往不是一種科學,反而更像藝術
Labels:
Investment,
Reading
Subscribe to:
Posts (Atom)