Total Pageviews
2016/03/31
2016/03/09
[Python] File I/O example
Example 1: Read file and print its content
Execution result:
Example 2: append some words at the end of file
Execution result:
Example 3. Apply with statement to open file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | import os """ 列印檔案內容 (print file content) """ def printFileContent(file): try: if(isFileExists("D:\\python\\txt\\" + file)==True): """ r stands for read """ data = open("D:\\python\\txt\\" + file, "r", encoding="utf-8") for each_line in data: print(each_line) else: print("查無檔案 : "+file) except IOError as err: print('IOError! ' + err.args[0]) finally: data.close() """" 檢查檔案是否存在 """ def isFileExists(file): if os.path.exists(file): return True else: return False |
Execution result:
1 2 3 4 5 6 7 | >>> import myUtil >>> myUtil.printFileContent("AJokeADay.txt") A rancher asked his veterinarian for some free advice. I have a horse that walks normally sometimes, and sometimes he limps. What shall I do? The Vet replied, The next time he walks normally, sell him. |
Example 2: append some words at the end of file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | import os """ 在檔案內容末端增加文字 (add words at the end of file content) """ def appendString(file, content): try: if(isFileExists("D:\\python\\txt\\" + file) == True): """ a stands for append """ data = open("D:\\python\\txt\\" + file, "a", encoding="utf-8") data.write("\n" + content) else: print("查無檔案:"+file) except IOError as err: print('IOError! ' + err.args[0]) finally: data.close() """ 列印檔案內容 (print file content) """ def printFileContent(file): try: if(isFileExists("D:\\python\\txt\\" + file)==True): """ r stands for read """ data = open("D:\\python\\txt\\" + file, "r", encoding="utf-8") for each_line in data: print(each_line) else: print("查無檔案 : "+file) except IOError as err: print('IOError! ' + err.args[0]) finally: data.close() """" 檢查檔案是否存在 """ def isFileExists(file): if os.path.exists(file): return True else: return False |
Execution result:
1 2 3 4 5 6 7 8 9 10 11 12 13 | >>> import time >>> addedContent = time.strftime("%Y/%m/%d")+" edited" >>> myUtil.appendString("AJokeADay.txt", addedContent) >>> >>> myUtil.printFileContent("AJokeADay.txt") A rancher asked his veterinarian for some free advice. I have a horse that walks normally sometimes, and sometimes he limps. What shall I do? The Vet replied, The next time he walks normally, sell him. 2015/12/23 edited >>> |
Example 3. Apply with statement to open file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | def printFileContent2(fileName): try: if(isFileExists("D:\\python\\txt\\" + fileName)==True): """ r stands for read """ with open("D:\\python\\txt\\" + fileName, "r", encoding="utf-8") as data: for each_line in data: print(each_line) else: print("查無檔案 : "+fileName) except IOError as err: print('IOError! ' + str(err)) finally: if 'data' in locals(): data.close() |
Labels:
Python
2016/03/08
[Python] IF...ELIF...ELSE Statements
Example
Assume I have four exam type, each type has its passing scores.
I will provide a method, as people fill in exam type and scores, it will you tell you pass the exam or not.
This example will demonstrate how to use if / elif / else in Python program:
Test:
Reference
[1] http://www.tutorialspoint.com/python/python_if_else.htm
Assume I have four exam type, each type has its passing scores.
I will provide a method, as people fill in exam type and scores, it will you tell you pass the exam or not.
This example will demonstrate how to use if / elif / else in Python program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | """ 判斷分數是否通過考試 """ def passExan(examType, scores): if examType == '1': if scores >= 252.79: print("恭喜您通過 專業1→營運職2 升等考試") else: print("很抱歉,您未通過 專業1→營運職2 升等考試") elif examType == '2': if scores >= 248.65: print("恭喜您通過 專業2→專業1 升等考試") else: print("很抱歉,您未通過 專業2→專業1 升等考試") elif examType == '3': if scores >= 260.79: print("恭喜您通過 專業3→專業2 升等考試") else: print("很抱歉,您未通過 專業3→專業2 升等考試") elif examType == '4': if scores >= 254.88: print("恭喜您通過 專業4→專業3 升等考試") else: print("很抱歉,您未通過 專業4→專業3 升等考試") else: print("查無此考試項目") |
Test:
1 2 3 4 5 6 7 | Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:38:48) [MSC v.1900 32 bit (Intel)] on win32 Type "copyright", "credits" or "license()" for more information. >>> import myUtil >>> myUtil.passExam("1", 270) 恭喜您通過 專業1→營運職2 升等考試 >>> myUtil.passExam("3", 180) 很抱歉,您未通過 專業3→專業2 升等考試 |
Reference
[1] http://www.tutorialspoint.com/python/python_if_else.htm
Labels:
Python
2016/03/07
[Python] Exception Handling
Problem
Here is my Python program:
The parameter in checkNum method only accept number data type. If I provide String data type...
How To
We can use try...except to do exception handling in Python program, and given more friendly exception message:
Test:
Here is my Python program:
1 2 3 4 5 6 | def checkNum(num): try: if(num>0): print("* number > 0 ( you input " + str(num) + " ).") else: print("* num < 0 ( you input " + str(num) + " ).") |
The parameter in checkNum method only accept number data type. If I provide String data type...
1 2 3 4 5 6 7 8 | >>> import myUtil >>> myUtil.checkNum("-20") Traceback (most recent call last): File "<pyshell#5>", line 1, in <module> myUtil.checkNum("-20") File "C:\Users\albert\AppData\Local\Programs\Python\Python35-32\lib\site-packages\myUtil.py", line 2, in checkNum if(num>0): TypeError: unorderable types: str() > int() |
How To
We can use try...except to do exception handling in Python program, and given more friendly exception message:
1 2 3 4 5 6 7 8 | def checkNum(num): try: if(num>0): print("* number > 0 ( you input " + str(num) + " ).") else: print("* num < 0 ( you input " + str(num) + " ).") except TypeError as err: print('只接受數字型態(Only Accept Number Data Type)! ' + err.args[0]) |
Test:
1 2 3 4 | >>> import myUtil >>> myUtil.checkNum("-20") 只接受數字型態(Only Accept Number Data Type)! unorderable types: str() > int() >>> |
Labels:
Python
2016/03/06
[Python] TypeError: Can't convert 'int' object to str implicitly
Problem
I have a Python program as bellows:
As I try to do test, it showed error message as following:
How To
You need to use str function to convert number to String
Test result:
Reference
[1] http://stackoverflow.com/questions/13654168/typeerror-cant-convert-int-object-to-str-implicitly
I have a Python program as bellows:
1 2 3 4 5 | def checkNum(num): if(num>0): print("* number > 0 ( you input " + num + " ).") else: print("* num < 0 ( you input " + num + " ).") |
As I try to do test, it showed error message as following:
1 2 3 4 5 6 7 8 | >>> import myUtil >>> myUtil.checkNum(10) Traceback (most recent call last): File "<pyshell#3>", line 1, in <module> myUtil.checkNum(10) File "C:\Users\albert\AppData\Local\Programs\Python\Python35-32\lib\site-packages\myUtil.py", line 3, in checkNum print('* number > 0 ( you input ' + num +' )') TypeError: Can't convert 'int' object to str implicitly |
How To
You need to use str function to convert number to String
1 2 3 4 5 | def checkNum(num): if(num>0): print("* number > 0 ( you input " + str(num) + " ).") else: print("* num < 0 ( you input " + str(num) + " ).") |
Test result:
1 2 3 4 5 6 | >>> import myUtil >>> myUtil.checkNum(89) * number > 0 ( you input 89 ). >>> myUtil.checkNum(-20) * num < 0 ( you input -20 ). >>> |
Reference
[1] http://stackoverflow.com/questions/13654168/typeerror-cant-convert-int-object-to-str-implicitly
Labels:
Python
2016/03/05
[Python] File I/O in Python : UnicodeDecodeError
Problem
I would like to open a text file and prints its content
But it throw this exception:
How To
Assign encoding to utf-8 as open this file.
I would like to open a text file and prints its content
1 2 3 4 5 | >>> import os >>> os.chdir ("D:\python") >>> data = open("txt\AJokeADay1.txt") >>> for eachLine in data : print(eachLine) |
But it throw this exception:
1 2 3 4 | Traceback (most recent call last): File "<pyshell#7>", line 1, in <module> for eachLine in data : UnicodeDecodeError: 'cp950' codec can't decode byte 0xe2 in position 70: illegal multibyte sequence |
How To
Assign encoding to utf-8 as open this file.
1 2 3 4 5 6 7 8 9 10 11 12 13 | >>> data = open("txt\AJokeADay1.txt", "r", encoding="utf-8") >>> for eachLine in data : print(eachLine) [In Office Jokes] A guy shows up late for work. The boss yells, ‘You should’ve been here at 8.30!’ He replies. ‘Why? What happened at 8.30?’ >>> data.close() |
Labels:
Python
2016/03/04
[Python] Module your Python Code
In Java, if some source code will be reused by others. We may create a utility class, if someone need this function, he/she can import this class and reuse this function. Do not need to reinvent the wheel.
Assume I have a simple Python code which named printLoop.py, the code is as bellows:
Supposed that many people may need this function to print the content of array. Therefore, you need to package this Python program as a module.
Step 1. Create a folder for my module which name printLoop

Step 2. Create a setup.py in printLoop folder
Step 3. Build distribution file
Step 4. Install the distribution file
Test.
1. Import printLoop module
2. Create a movie list
3. utilize printLoop.printMovies to print movie list
Assume I have a simple Python code which named printLoop.py, the code is as bellows:
1 2 3 4 | """ print movie list""" def printMovies(movies): for movie in movies : print(movie) |
Supposed that many people may need this function to print the content of array. Therefore, you need to package this Python program as a module.
Step 1. Create a folder for my module which name printLoop

Step 2. Create a setup.py in printLoop folder
1 2 3 4 5 6 7 8 9 10 | from distutils.core import setup setup( name = 'printLoop', version = '1.0.0', py_modules = ['printLoop'], author = 'albert', author_email = 'xxx@gmail.com', url = 'http://xxx.com', description = 'simple example for building module', ) |
Step 3. Build distribution file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | D:\python\printLoop>python setup.py sdist running sdist running check warning: sdist: manifest template 'MANIFEST.in' does not exist (using default file list) warning: sdist: standard file not found: should have one of README, README.txt writing manifest file 'MANIFEST' creating printLoop-1.0.0 making hard links in printLoop-1.0.0... hard linking printLoop.py -> printLoop-1.0.0 hard linking setup.py -> printLoop-1.0.0 creating 'dist\printLoop-1.0.0.zip' and adding 'printLoop-1.0.0' to it adding 'printLoop-1.0.0\PKG-INFO' adding 'printLoop-1.0.0\printLoop.py' adding 'printLoop-1.0.0\setup.py' removing 'printLoop-1.0.0' (and everything under it) |
Step 4. Install the distribution file
1 2 3 4 5 6 7 8 9 10 11 12 13 | D:\python\printLoop>python setup.py install running install running build running build_py creating build creating build\lib copying printLoop.py -> build\lib running install_lib copying build\lib\printLoop.py -> C:\Users\albert\AppData\Local\Programs\Python\Python35-32\Lib\site-packages byte-compiling C:\Users\albert\AppData\Local\Programs\Python\Python35-32\Lib\site-packages\printLoop.py to printLoop.cpython-35.pyc running install_egg_info Removing C:\Users\albert\AppData\Local\Programs\Python\Python35-32\Lib\site-packages\printLoop-1.0.0-py3.5.egg-info Writing C:\Users\albert\AppData\Local\Programs\Python\Python35-32\Lib\site-packages\printLoop-1.0.0-py3.5.egg-info |
Test.
1. Import printLoop module
2. Create a movie list
3. utilize printLoop.printMovies to print movie list
1 2 3 4 5 6 7 8 9 | Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:38:48) [MSC v.1900 32 bit (Intel)] on win32 Type "copyright", "credits" or "license()" for more information. >>> import printLoop >>> movies = ["Inside Out", "The Intern", "Our Brand is Crisis"] >>> printLoop.printMovies(movies) Inside Out The Intern Our Brand is Crisis >>> |
Labels:
Python
2016/03/03
[Python] How to Change Working Directory in IDLE
Problem
As I open IDLE, how do I know my current working directory? And how to change to another working directory?
How To
1. you need to import os module first
2. use os.getcwd() to get current working directory
3. use os.chdir("XXX") to change working directory
Reference
[1] http://stackoverflow.com/questions/15821121/whats-the-working-directory-when-using-idle
As I open IDLE, how do I know my current working directory? And how to change to another working directory?
How To
1. you need to import os module first
2. use os.getcwd() to get current working directory
3. use os.chdir("XXX") to change working directory
1 2 3 4 5 6 7 8 9 | Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:38:48) [MSC v.1900 32 bit (Intel)] on win32 Type "copyright", "credits" or "license()" for more information. >>> import os >>> os.getcwd() 'C:\\Users\\albert\\AppData\\Local\\Programs\\Python\\Python35-32' >>> os.chdir("D:\python") >>> os.getcwd() 'D:\\python' >>> |
Reference
[1] http://stackoverflow.com/questions/15821121/whats-the-working-directory-when-using-idle
Labels:
Python
2016/03/02
[Python] Set Up Python Environment
Step 1. Download Python from https://www.python.org/downloads/windows/
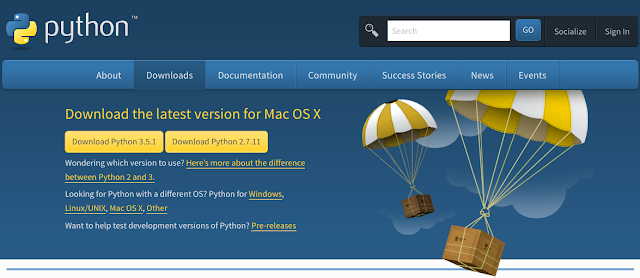
Step 2. Install Python
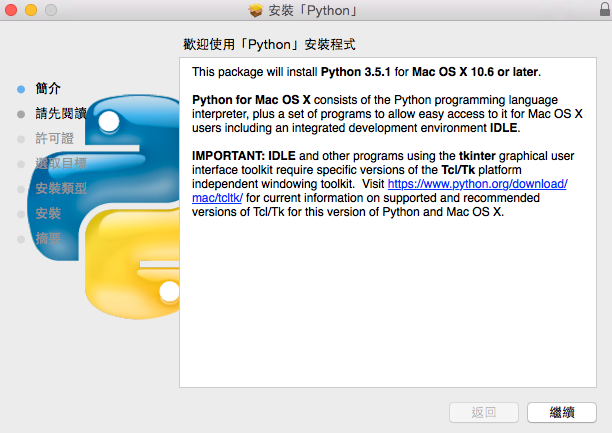
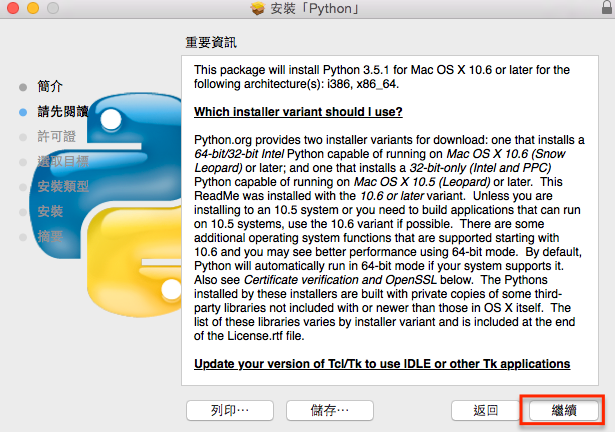
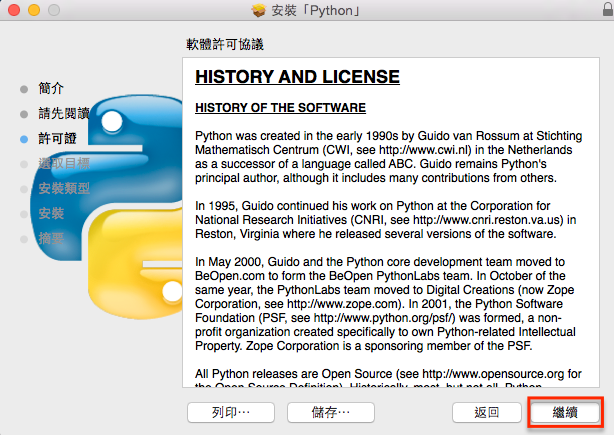
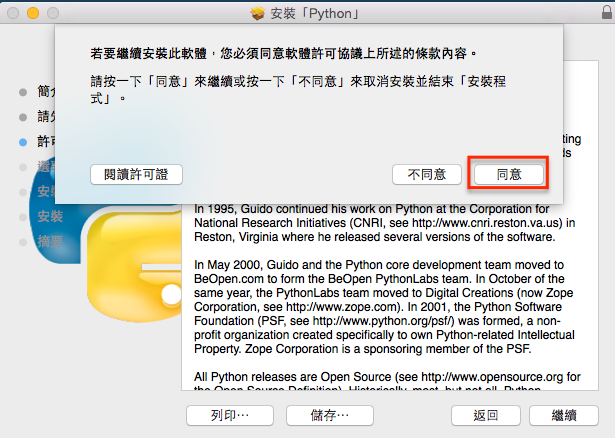
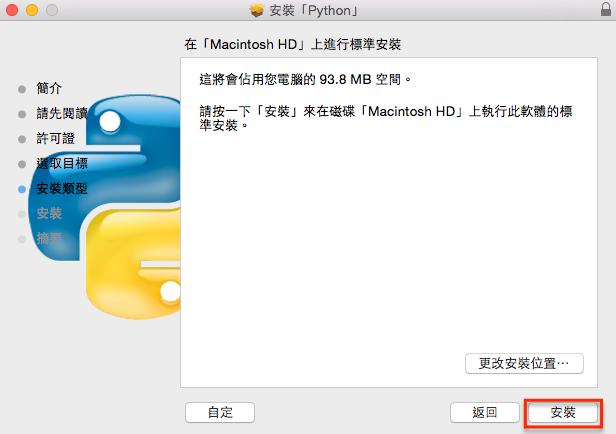
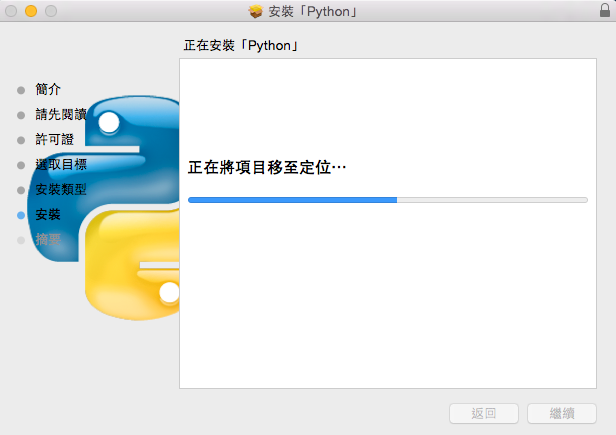
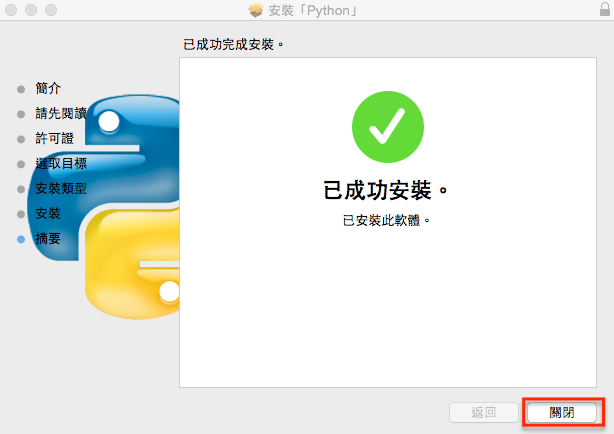
Step 3. If you are Windows users, you need to configure Python installation path and Scripts path to path (environment variables)
i.e. C:\Python35-32\ and C:\Python35-32\Scripts
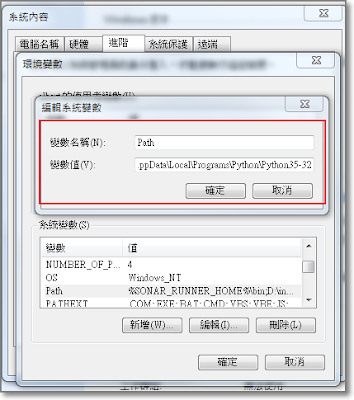
If you are Mac user, you can ignore this step.
Step 4. Open command prompt, and type in python3 -V to do verification.

Write a first and simple Python program
Then you can use IDLE to help you learn Python
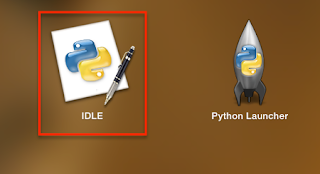
IDLE knows all about Python syntax
Ex1. Use terminal
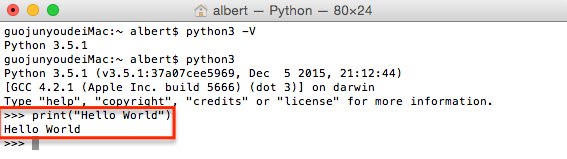
Ex2. Use IDLE
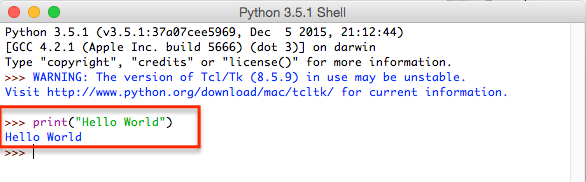
IDEL also offers "code completion" when you use build-in functions, ex. print()
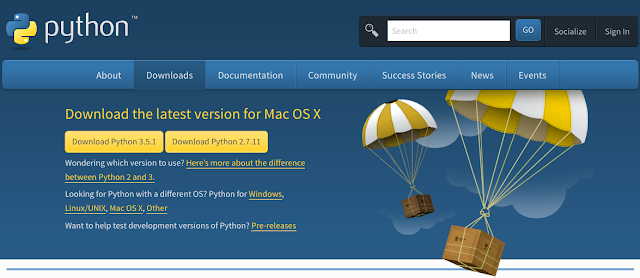
Step 2. Install Python
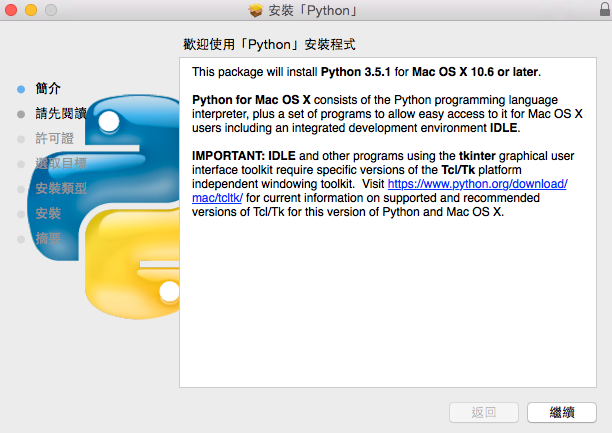
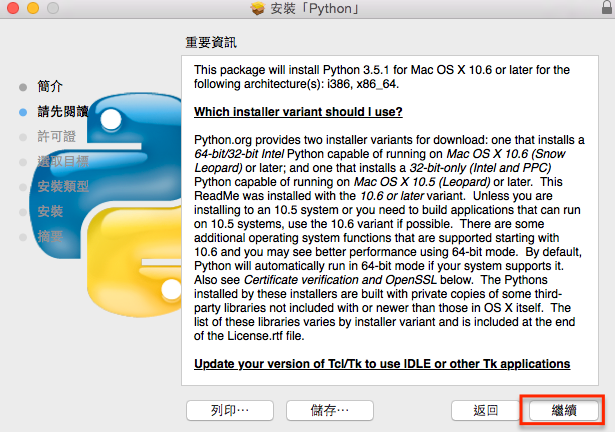
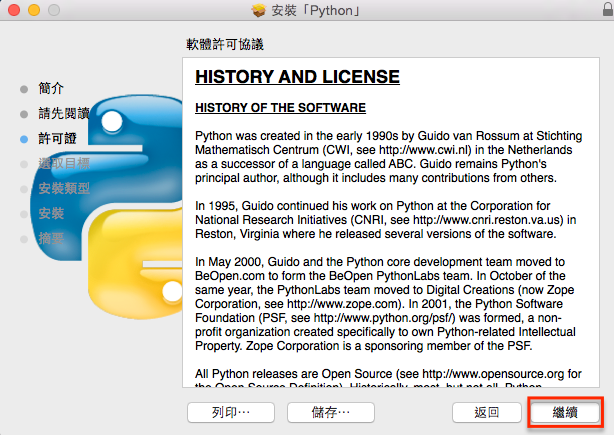
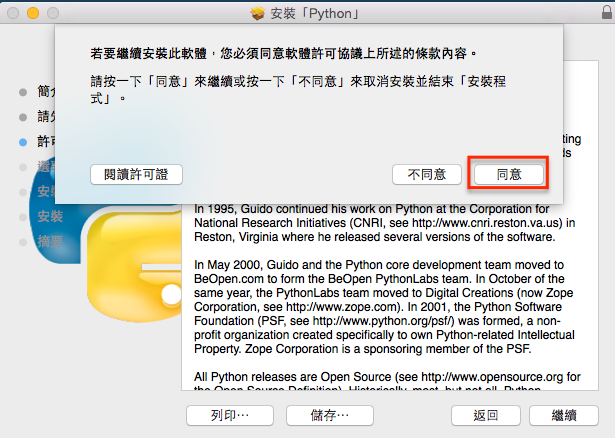
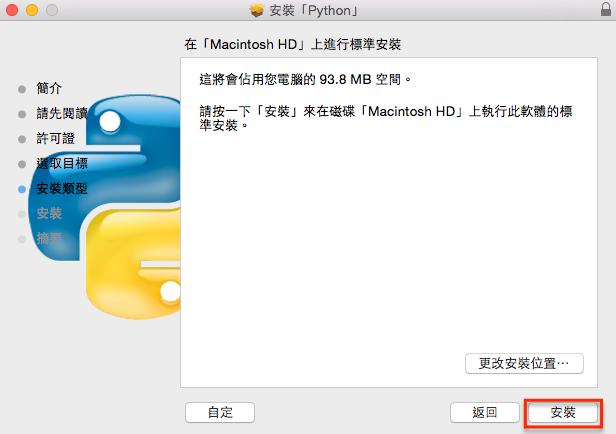
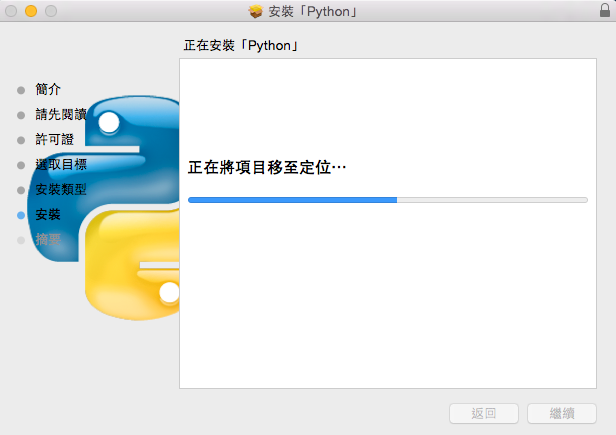
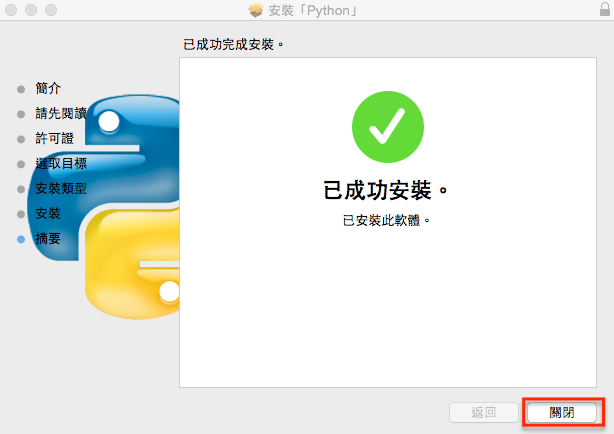
Step 3. If you are Windows users, you need to configure Python installation path and Scripts path to path (environment variables)
i.e. C:\Python35-32\ and C:\Python35-32\Scripts
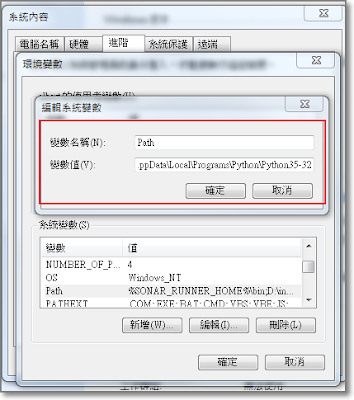
If you are Mac user, you can ignore this step.
Step 4. Open command prompt, and type in python3 -V to do verification.

Write a first and simple Python program
1 2 3 4 | # encoding: utf-8 print("Hello, Python") print("哈囉,派森") |
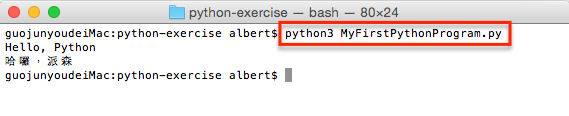
Then you can use IDLE to help you learn Python
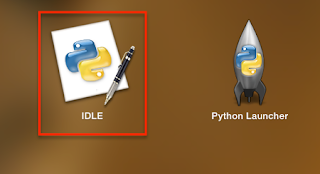
IDLE knows all about Python syntax
Ex1. Use terminal
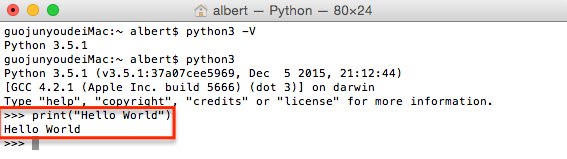
Ex2. Use IDLE
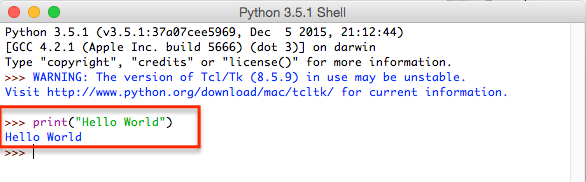
IDEL also offers "code completion" when you use build-in functions, ex. print()
Labels:
Python
2016/03/01
[閱讀筆記] 富爸爸,窮爸爸 (Part 2)
- 風險無所不在,要學會駕馭風險,而不是一昧低迴避風險
- 工人付出最高限度的努力工作以避免被解雇,而雇主提供最低額度的工資以防止工人辭職
- 麥當勞擁有一套出色的商務體系。許多才華洋溢的人之所以貧窮,是因為他們只專心做好漢堡,而對如何運作商務體系卻所知甚少。
- 成功所必要的管理素質包括:
- 對現金流的管理
- 對系統(包括你本人、時間及家庭)的管理
- 對人員的管理
- 人們即便是經過學習,掌握財務知,但是在通往財務自由的道路上人面臨許多困難,包含:恐懼心理、憤世嫉俗、懶惰、不良習慣、自負
- 在投資活動中,從沒遇過從未損失的富人,但常遇到從未損失過一毛的窮人
- 如果你討厭冒險,對金錢損失感到擔心,那就早點動手累積屬於妳的金錢
- 在財務上不能獲得成功的最大原因是大部份的人的作法太安全。人們因為太害怕失敗,所以才會失敗
- 人人都想上天堂,卻沒有人想死。可是不死怎麼上天堂。這就如同大部份人夢想發財,但卻害怕在投資過程損失金錢,所以她們永遠進不了天堂
- 洛克斐洛曾說:我總是試圖將每一次災難轉換成機會
- 90%的美國公民財務困難的主要原因在於他們是為了避免損失而理財,而不是為了營利而理財
- 失敗會激勵勝利者,也會撃垮失敗者。勝利意味著不害怕失敗,他們了解自己,也討厭失敗,但失敗會激勵他們做的更好
- 如果妳沒有什麼資金又有致富的願望,妳就必須集中精力,把妳僅有的雞蛋放在較少的籃子(當然你還要確信籃子的結實程度),不要把很少的雞蛋放在許多籃子裡
- 如果妳不願失敗,那就安全的投資;如果損失會讓你元氣大傷,那就安妥一點,去做一個平衡的投資。但以安全的方式進行投資,就要儘早起步,儘早開始累積妳的雞蛋,因為以這種方式需要大量的時間
- 未經證實的懷疑和恐懼會產生憤世嫉俗者。憤世嫉俗者抱怨現實,從來不會贏;成功者分析現實,想辦法贏
- 我們用錢的習慣決定我們是什麼類型的人,有的人之所以貧窮是因為他們有著不良的用錢習慣
- 聰明 + 傲慢 = 無知
- 如果你想駕駛飛機,你應該去聽有關飛行原理的課程,而不是直接坐進駕駛艙。妳買了一兩套房地產,不會讓你成為房地產專家。你應該花時間投資你最重要的資產 - 頭腦
- 累積財富的過程,最困難的事情莫過於堅持自己的選擇而不盲目從眾
- 是否缺乏自律,是將富人與窮人區分開來的首要因素。生活之所以推著你轉,不是因為驅使你的人很厲害,而是因為你個人缺乏自我控制和紀律
- 開創你自己的事業需具備三個重要的管理技能:現金流量管理、人事管理、個人時間管理
- 不要背負數額過大的債務包袱,要使自己的支出保持低水平。先增加自己的資產,然後再用自己的資產中產生的現金流購買大房子、大車子
- 當你資金短缺時,去承受外在壓力而不要動用你的儲蓄或投資,利用這種壓力去激發你的財務天賦,想辦法掙出更多的錢,然後再支付帳單
- 窮人有個不好的習慣,就是隨便動用儲蓄,富人知道儲蓄只能用於創造更多的錢,而不是用來支付帳單
- 人事管理是要能夠管理在某些技術領域比你聰明的人,而不是只會管理沒有自己聰明的人或能力沒有自己強的人
- 最容易的道路往往會越走越艱難,而艱難的道路往往會越走越輕鬆
- 如果你的財務智商很低,金錢就會比你更精明,他會從你身上溜走。如果你沒有金錢精明,你將為他工作一生。要成為金錢的主人,你要比金錢更精明,讓金錢為你工作,而不是成為它的奴隸,這就是財務智商
- 行動吧!行動者總會擊敗不行動者
- 思考是一種思想,你應該思考致富,而不是努力工作致富。讓金錢為你辛勤工作,你的生活將會變得更輕鬆、更幸福
- 上天賜與每個人兩樣禮物:思想與時間。隨著每一張鈔票流入你的手中,愚蠢地用掉它,你就選擇了貧困;把錢用在負債專案上,你就會進入中產階級;投資於你的頭腦,學習如何獲得資產,財富將成為你的目標和你的未來
- 為你的財務負起責任或一生只聽從別人的命令,你要嘛是金錢的主人,亦或是金錢的奴隸
Labels:
Investment,
Reading
Subscribe to:
Posts (Atom)