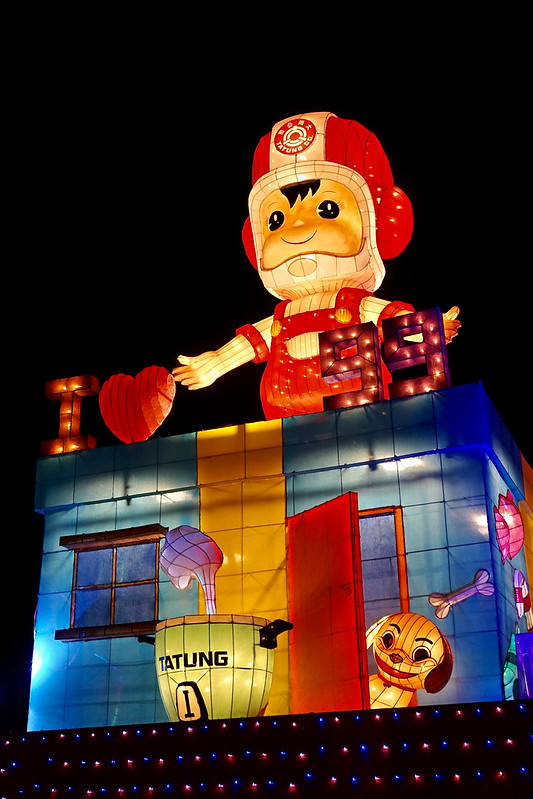

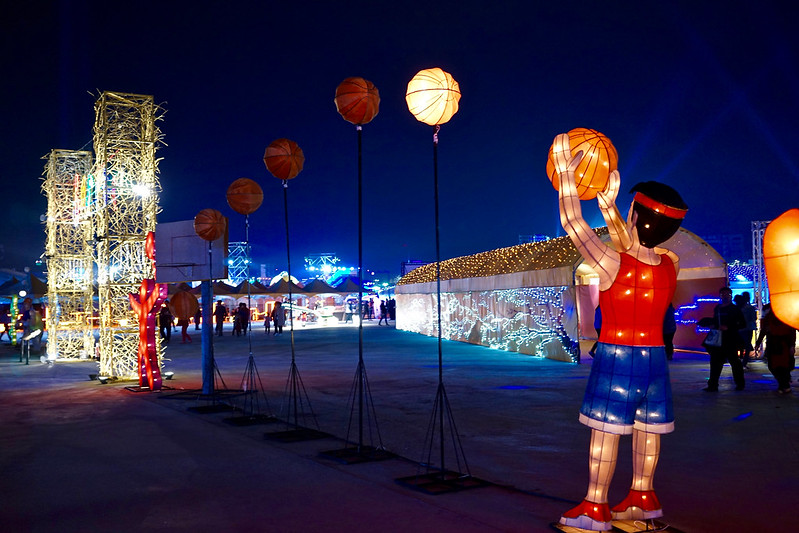



1 2 | String content = report.getContent();
out.print(content);
|
1 2 3 4 5 | <dependency> <groupId>org.owasp.encoder</groupId> <artifactId>encoder</artifactId> <version>1.2.1</version> </dependency> |
1 2 3 4 5 | <%@ page import="org.owasp.encoder.Encode"%> String content = report.getContent(acct_no,schema+"://"+server+":"+port); out.print(Encode.forHtml(content)); |
1 2 3 4 5 6 7 8 9 10 11 | { "id": 1, "name": "Albert", "email": "albert@gmail.com", "phone": "0900123456", "address": { "streetAddress": "信義區信義路五段7號", "city": "台北市", "zipCode": "110" } } |
1 2 3 4 5 6 7 8 9 10 | --- id: 1 name: "Albert" email: "albert@gmail.com" phone: "0900123456" address: streetAddress: "信義區信義路五段7號" city: "台北市" zipCode: "110" |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.9.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.9.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.9.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-yaml</artifactId> <version>2.9.3</version> </dependency> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | package test.albert.jackson; import java.io.IOException; import com.fasterxml.jackson.databind.JsonNode; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.dataformat.yaml.YAMLMapper; import lombok.extern.slf4j.Slf4j; @Slf4j public class JacksonTest { public static void main(String[] args) throws IOException { Address address = Address.builder().zipCode("110").city("台北市").streetAddress("信義區信義路五段7號").build(); Employee albert = Employee.builder().id(1).name("Albert").email("albert@gmail.com").phone("0900123456") .address(address).build(); ObjectMapper mapper = new ObjectMapper(); // convert object to JSON String json = mapper.writeValueAsString(albert); log.debug(json); // convert JSON to YAML JsonNode jsonNode = mapper.readTree(json); String yaml = new YAMLMapper().writeValueAsString(jsonNode); log.debug("yaml = \n " + yaml); } } |
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'liquibase' defined in class path resource [org/springframework/boot/autoconfigure/liquibase/LiquibaseAutoConfiguration$LiquibaseConfiguration.class]: Invocation of init method failed; nested exception is liquibase.exception.ValidationFailedException: Validation Failed: 1 change sets check sum classpath:/db/changelog/db.changelog-master.yaml::1::albert was: 7:6e574692a29b35c6788f06c99fe2eecc but is now: 7:c5994a0c5ad9c2ba211a4f8e3ced2ec9
delete from databasechangelog
java.lang.UnsupportedClassVersionError: org/apache/tools/ant/launch/Launcher : Unsupported major.minor version 52.0
mvn -o -Dmaven.repo.local="C:/Users/albert/.m2" clean install -Dmaven.test.skip=trueDuring the build process, Maven still check for updated for certain artifacts even in offline mode. How to avoid it?
select to_char(now(), 'YYYYMMDD')
select LPAD(nextval('loan_sequence')::text, 10, '0')
ALTER TABLE loan_main ALTER COLUMN loan_id SET DEFAULT ('LN_' || (to_char(now(), 'YYYYMMDD')) || '_' || LPAD(nextval('loan_sequence')::text, 10, '0'));
<!-- validation start --> <dependency> <groupId>javax.validation</groupId> <artifactId>validation-api</artifactId> <version>2.0.0.Final</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> <version>6.0.2.Final</version> </dependency> <dependency> <groupId>org.glassfish</groupId> <artifactId>javax.el</artifactId> <version>3.0.1-b08</version> </dependency> <!-- validation end --> <!-- DI start --> <dependency> <groupId>com.google.inject</groupId> <artifactId>guice</artifactId> <version>4.1.0</version> </dependency> <!-- DI end --> <!-- lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>$1.16.18</version> <scope>provided</scope> </dependency> <dependency> <groupId>com.google.guava</groupId> <artifactId>guava</artifactId> <version>21.0</version> </dependency>
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | package albert.practice.validation; import java.util.Date; import javax.validation.constraints.Email; import javax.validation.constraints.NotEmpty; import javax.validation.constraints.NotNull; import javax.validation.constraints.Past; import org.hibernate.validator.constraints.Range; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import lombok.ToString; @AllArgsConstructor @NoArgsConstructor @ToString @Data public class Customer { public static enum Gender { MALE, FEMALE; } @NotNull(message = "顧客編號不可為 null") private Long id; @NotEmpty(message = "姓名不可為空") private String name; @NotNull(message = "性別不可為空") private Gender gender; @Range(min = 18, max = 60, message = "年齡必須介於 18 到 60 歲之間") private Integer age; @NotEmpty(message = "Email 不可為空") @Email(message = "Email 格式不合法") private String email; @Past(message = "生日必須要今天以前") private Date dateOfBirth; } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | package albert.practice.validation; import java.util.ArrayList; import java.util.List; import java.util.Set; import javax.validation.ConstraintViolation; import javax.validation.Validation; import javax.validation.Validator; import com.google.common.base.Joiner; import com.google.common.base.Strings; import com.google.inject.Singleton; import lombok.extern.slf4j.Slf4j; @Slf4j @Singleton public class CustomerService { public void create(Customer customer) { validate(customer); } public static void validate(Object obj) { List<String> errors = new ArrayList<>(); Validator validator = Validation.buildDefaultValidatorFactory().getValidator(); Set<ConstraintViolation<Object>> violations = validator.validate(obj); for (ConstraintViolation<Object> violation : violations) { errors.add(violation.getMessage()); } String completeErrorMsg = Joiner.on("\n").join(errors); if (!Strings.isNullOrEmpty(completeErrorMsg)) { throw new IllegalArgumentException(completeErrorMsg); } } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | package albert.practice.validation; import com.google.inject.Guice; import com.google.inject.Inject; public class CustomerResource { @Inject private CustomerService service; public static void main(String[] args) { Customer customer = new Customer(); customer.setId(null); customer.setAge(12); customer.setEmail("test@gmail."); new CustomerResource().createCustomer(customer); } public void createCustomer(Customer customer){ service = Guice.createInjector().getInstance(CustomerService.class); service.create(customer); } } |
1 2 3 4 5 6 7 8 9 | Exception in thread "main" java.lang.IllegalArgumentException: 性別不可為空 年齡必須介於 18 到 60 歲之間 Email 格式不合法 顧客編號不可為 null 姓名不可為空 at albert.practice.validation.CustomerService.validate(CustomerService.java:35) at albert.practice.validation.CustomerService.create(CustomerService.java:20) at albert.practice.validation.CustomerResource.createCustomer(CustomerResource.java:22) at albert.practice.validation.CustomerResource.main(CustomerResource.java:17) |
Validator validator = Validation.buildDefaultValidatorFactory().getValidator();
Exception in thread "main" javax.validation.ValidationException: HV000183: Unable to initialize 'javax.el.ExpressionFactory'. Check that you have the EL dependencies on the classpath, or use ParameterMessageInterpolator instead at org.hibernate.validator.messageinterpolation.ResourceBundleMessageInterpolator.buildExpressionFactory(ResourceBundleMessageInterpolator.java:115) at org.hibernate.validator.messageinterpolation.ResourceBundleMessageInterpolator.<init>(ResourceBundleMessageInterpolator.java:46) at org.hibernate.validator.internal.engine.ConfigurationImpl.getDefaultMessageInterpolator(ConfigurationImpl.java:420) at org.hibernate.validator.internal.engine.ConfigurationImpl.getDefaultMessageInterpolatorConfiguredWithClassLoader(ConfigurationImpl.java:596) at org.hibernate.validator.internal.engine.ConfigurationImpl.getMessageInterpolator(ConfigurationImpl.java:355) at org.hibernate.validator.internal.engine.ValidatorFactoryImpl.<init>(ValidatorFactoryImpl.java:149) at org.hibernate.validator.HibernateValidator.buildValidatorFactory(HibernateValidator.java:38) at org.hibernate.validator.internal.engine.ConfigurationImpl.buildValidatorFactory(ConfigurationImpl.java:322) at javax.validation.Validation.buildDefaultValidatorFactory(Validation.java:103) at albert.practice.validation.CustomerService.validate(CustomerService.java:38) at albert.practice.validation.CustomerService.create(CustomerService.java:21) at albert.practice.validation.CustomerService.main(CustomerService.java:32) Caused by: java.lang.NoClassDefFoundError: javax/el/ELManager at org.hibernate.validator.messageinterpolation.ResourceBundleMessageInterpolator.buildExpressionFactory(ResourceBundleMessageInterpolator.java:107) ... 11 more Caused by: java.lang.ClassNotFoundException: javax.el.ELManager at java.net.URLClassLoader.findClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) ... 12 more
<dependency> <groupId>org.glassfish</groupId> <artifactId>javax.el</artifactId> <version>3.0.1-b08</version> </dependency>
1 2 3 4 5 | <dependency> <groupId>com.google.inject</groupId> <artifactId>guice</artifactId> <version>4.1.0</version> </dependency> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | package albert.practice.guice; import java.util.List; import com.google.common.collect.ImmutableList; import com.google.inject.Singleton; import lombok.extern.slf4j.Slf4j; @Slf4j @Singleton public class ContactService { public List<Contact> getContacts(String id) { log.debug("person id is " + id); return ImmutableList.of(new Contact("Mandy", "0912111111"), new Contact("Dad", "0911111111"), new Contact("Mom", "0922222222")); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | package albert.practice.guice; import java.util.List; import com.google.inject.Inject; public class PersonResource { @Inject private ContactService contactService; public List<Contact> getContacts(String id) { return contactService.getContacts(id); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | package albert.practice.guice; import static org.junit.Assert.assertEquals; import java.util.List; import org.junit.Before; import org.junit.Test; import com.google.inject.Guice; import lombok.extern.slf4j.Slf4j; @Slf4j public class PersonResourceTest { private PersonResource personResouce; @Before public void setup() { personResouce = Guice.createInjector().getInstance(PersonResource.class); } @Test public void testGetContacts() { List<Contact> contacts = personResouce.getContacts("123"); contacts.stream().forEach(c -> log.debug(c.toString())); assertEquals(3, contacts.size()); } } |