


Piccadilly Circus



The Roman Baths




The circle


Notting Hill


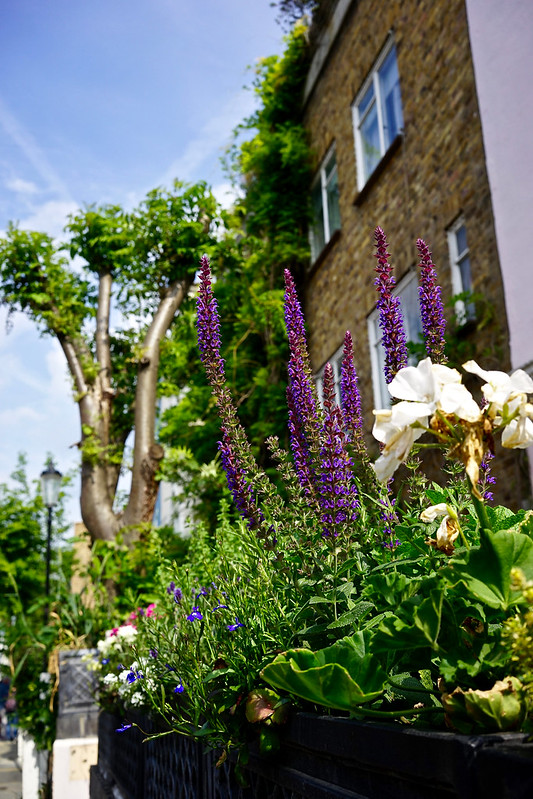
Harrods

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | package albert.practice.designpattern.builder; import java.util.regex.Pattern; import lombok.Getter; import lombok.ToString; @Getter @ToString public class User { private final String firstName; private final String lastName; private final Integer age; private final String phone; private final String email; private User(UserBuilder builder) { this.firstName = builder.firstName; this.lastName = builder.lastName; this.age = builder.age; this.phone = builder.phone; this.email = builder.email; } public static class UserBuilder { private String firstName; private String lastName; private Integer age; private String phone; private String email; public UserBuilder firstName(String firstName) { this.firstName = firstName; return this; } public UserBuilder lastName(String lastName) { this.lastName = lastName; return this; } public UserBuilder age(Integer age) { this.age = age; return this; } public UserBuilder phone(String phone) { this.phone = phone; return this; } public UserBuilder email(String email) { this.email = email; return this; } public User build() { User user = new User(this); // Do some basic validations to check if (!isEmailValid(user.getEmail())) { throw new RuntimeException(user.getEmail() + " is invalid Email format!"); } return user; } public Boolean isEmailValid(String email) { Boolean isValid = Boolean.FALSE; Pattern EMAIL_PATTERN = Pattern.compile("^\\w+\\.*\\w+@(\\w+\\.){1,5}[a-zA-Z]{2,3}$"); if (EMAIL_PATTERN.matcher(email).matches()) { isValid = Boolean.TRUE; } return isValid; } } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | package albert.practice.designpattern.builder; import albert.practice.designpattern.builder.User.UserBuilder; import lombok.extern.slf4j.Slf4j; @Slf4j public class BuilderTest { public static void main(String[] args) { User albert = new UserBuilder() .lastName("Kuo") .firstName("Albert") .phone("1234567890") .age(30) .email("test@gmail.com") .build(); log.debug(" user1 = " + albert.toString()); User mandy = new UserBuilder() .lastName("Yeh") .firstName("Mandy") .email("yeh@gmail.com") .build(); log.debug(" user2 = " + mandy.toString()); } } |
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-csv</artifactId> <version>1.4</version> </dependency>
package albert.practice.csv; import java.io.Serializable; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import lombok.ToString; @Data @AllArgsConstructor @NoArgsConstructor @ToString public class OpcVo implements Serializable { private Integer ns; private String identifier; }
package albert.practice.csv; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import org.apache.commons.csv.CSVFormat; import org.apache.commons.csv.CSVParser; import org.apache.commons.csv.CSVPrinter; import org.apache.commons.csv.CSVRecord; import lombok.extern.slf4j.Slf4j; @Slf4j public class CSVTest { // Delimiter used in CSV file private static final String NEW_LINE_SEPARATOR = "\n"; // CSV file header private static final String[] FILE_HEADER = {"ns", "identifier"}; // OpcVo attributes private static final String NS = "ns"; private static final String ID = "identifier"; public static void main(String[] args) throws IOException { String csvDirectory = "D:/work/AOCS/csv/"; String csvFileName = "test.csv"; CSVTest test = new CSVTest(); // test.writeToCSV(csvFolder.concat(csvFile)); List<OpcVo> opcs = test.readFromCsv(csvDirectory.concat(csvFileName)); opcs.forEach(opc -> log.debug(opc.toString())); } public void writeToCSV(String fileName) throws IOException { List<OpcVo> opcs = getDummyData(); // Create the CSVFormat object with "\n" as a record delimiter CSVFormat csvFileFormat = CSVFormat.DEFAULT.withRecordSeparator(NEW_LINE_SEPARATOR); try (FileWriter fileWriter = new FileWriter(fileName); CSVPrinter csvFilePrinter = new CSVPrinter(fileWriter, csvFileFormat);) { // Create CSV file header csvFilePrinter.printRecord(FILE_HEADER); // Write a new OpcVo object list to the CSV file for (OpcVo opc : opcs) { List dataRecord = new ArrayList<>(); dataRecord.add(String.valueOf(opc.getNs())); dataRecord.add(opc.getIdentifier()); csvFilePrinter.printRecord(dataRecord); } } catch (IOException e) { throw e; } } public List<OpcVo> readFromCsv(String fileName) throws IOException { // Create a new list of OpcVo to be filled by CSV file data List<OpcVo> result = new ArrayList<>(); // Create the CSVFormat object with the header mapping CSVFormat csvFileFormat = CSVFormat.DEFAULT.withHeader(FILE_HEADER); try (FileReader fileReader = new FileReader(fileName); CSVParser csvFileParser = new CSVParser(fileReader, csvFileFormat);) { List dataRecord = new ArrayList<>(); // Get a list of CSV file records List<CSVRecord> csvRecords = csvFileParser.getRecords(); for (int i = 1; i < csvRecords.size(); i++) { //Create a new OpcVo object and fill this data CSVRecord record = csvRecords.get(i); String ns = record.get(NS); String identifier = record.get(ID); result.add(new OpcVo(Integer.valueOf(ns), identifier)); } } catch (IOException e) { throw e; } return result; } private List<OpcVo> getDummyData() { OpcVo opc1 = new OpcVo(2, "Channel1.Device1.FireZone_01"); OpcVo opc2 = new OpcVo(2, "Channel1.Device1.EmergencyPushButton_01"); OpcVo opc3 = new OpcVo(2, "Channel1.Device1.EmergencyExitWindow_01"); OpcVo opc4 = new OpcVo(2, "Channel1.Device1.EmergencyExit_01"); return Arrays.asList(opc1, opc2, opc3, opc4); } }