
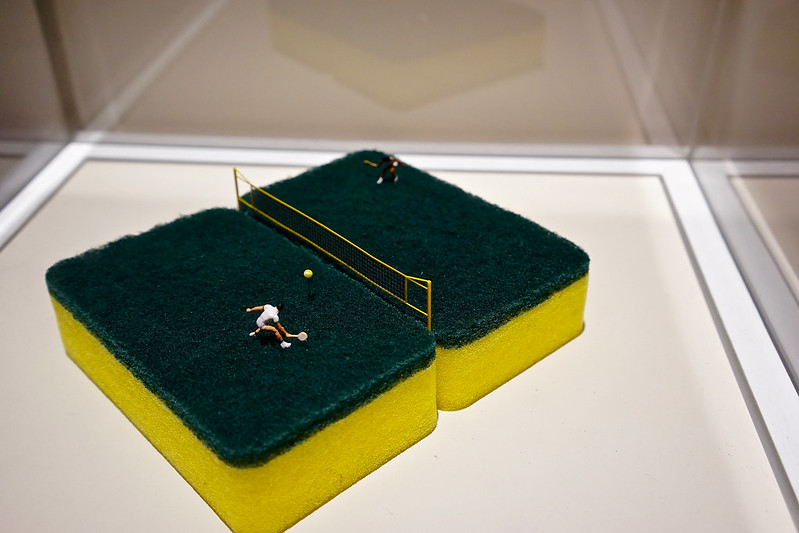


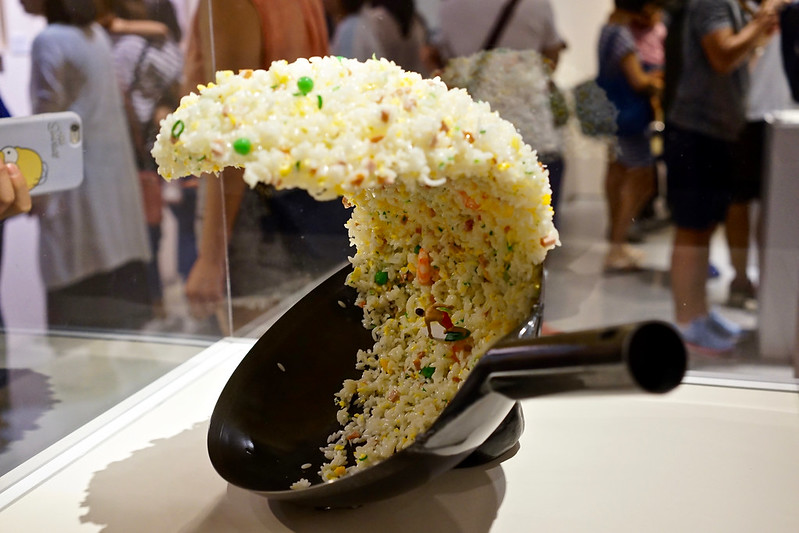
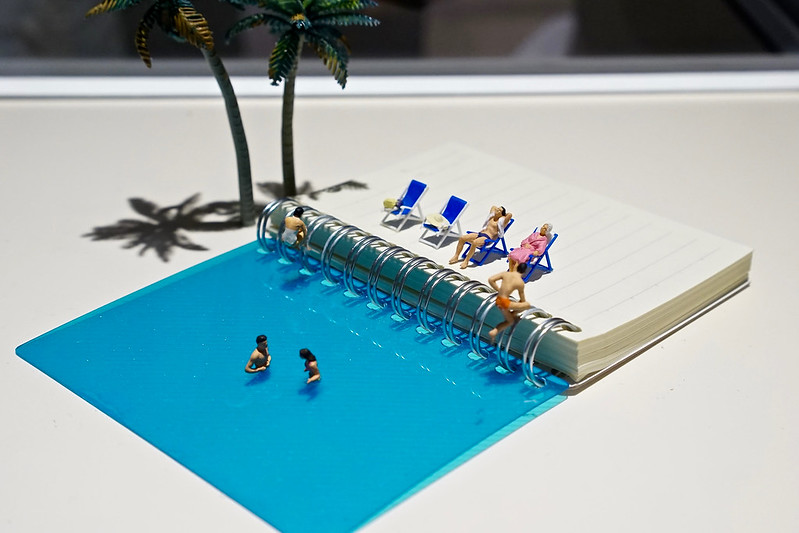

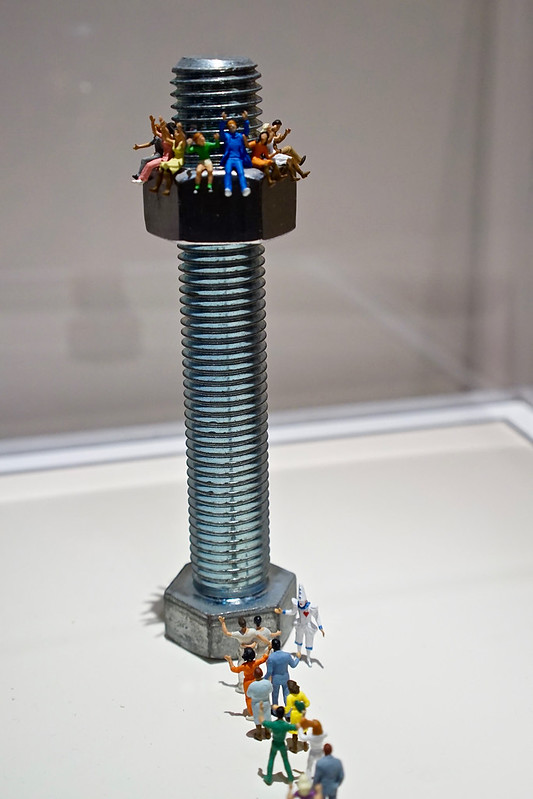
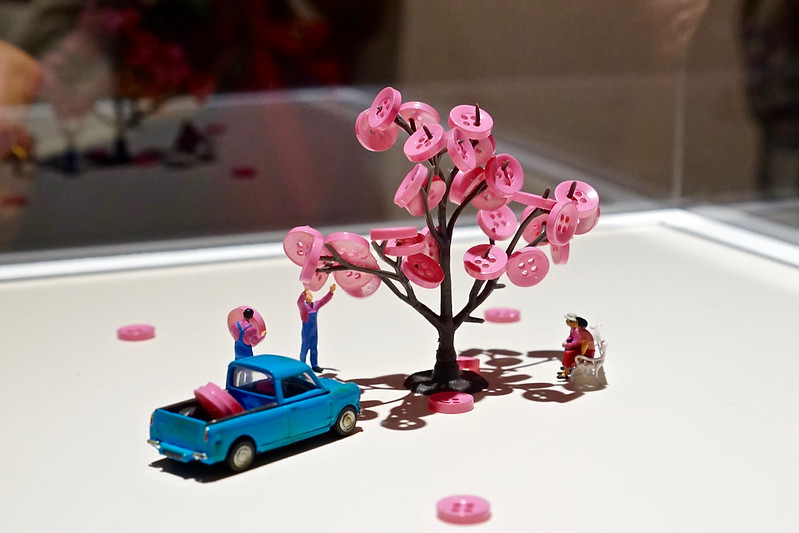

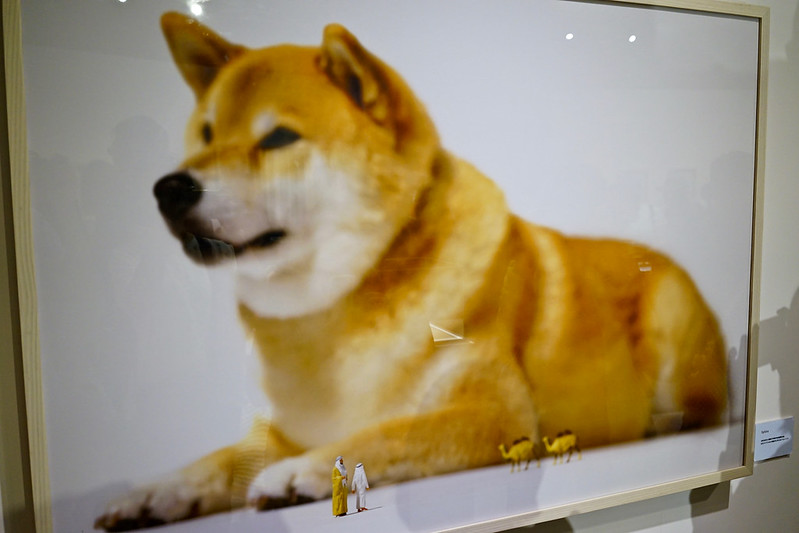
DELETE FROM test_table where id between 1 and 500000;
The transaction log for the database is full.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 | -- delete rownumber from 1 to 100000 DELETE FROM test_table WHERE id IN ( SELECT id FROM (SELECT id, row_num() over(ORDER BY id) AS rownumber FROM test_table) WHERE rownumber BETWEEN 1 AND 100000 ); -- delete rownumber from 100001 to 200000 DELETE FROM test_table WHERE id IN ( SELECT id FROM (SELECT id, row_num() over(ORDER BY id) AS rownumber FROM test_table) WHERE rownumber BETWEEN 100001 AND 200000 ); -- delete rownumber from 200001 to 300000 DELETE FROM test_table WHERE id IN ( SELECT id FROM (SELECT id, row_num() over(ORDER BY id) AS rownumber FROM test_table) WHERE rownumber BETWEEN 200001 AND 300000 ); -- delete rownumber from 300001 to 400000 DELETE FROM test_table WHERE id IN ( SELECT id FROM (SELECT id, row_num() over(ORDER BY id) AS rownumber FROM test_table) WHERE rownumber BETWEEN 300001 AND 400000 ); -- delete rownumber from 400001 to 500000 DELETE FROM test_table WHERE id IN ( SELECT id FROM (SELECT id, row_num() over(ORDER BY id) AS rownumber FROM test_table) WHERE rownumber BETWEEN 400001 AND 500000 ); |
1 2 3 4 5 6 7 8 | try { // Invokes any service published on the server with the given input arguments. IData iData = Service.doInvoke("test.work", "Ping_Server", IDataFactory.create()); // Returns a String representation of the value at the specified key. String isAlive = IDataUtil.getString(iData.getCursor(), "isAlive"); } catch (Exception e1) { e1.printStackTrace(); } |
1 2 3 4 5 6 7 8 9 10 11 | try { // Invokes any service published on the server as a thread ServiceThread serviceThread = Service.doThreadInvoke("test.work", "Ping_Server", session, IDataFactory.create()); // Returns the pipeline from the service running in this service thread. IData iData = serviceThread.getIData(); // Returns a String representation of the value at the specified key. String isAlive = IDataUtil.getString(iData.getCursor(), "isAlive"); loggerAsync(session, " is Alive = " + isAlive); } catch (Exception e) { e.printStackTrace(); } |
Session session = Service.getSession(); Service.doThreadInvoke("test.work", "Test_Flow", session, IDataFactory.create());
Session session = Service.getSession(); IData input = IDataFactory.create(); IDataCursor inputCursor = input.getCursor(); IDataUtil.put(inputCursor, "input", " test test "); inputCursor.destroy(); Service.doThreadInvoke("test.work", "Test_Flow", session, input);
Service.doInvoke("test.work", "Test_Flow", IDataFactory.create());
IData input = IDataFactory.create(); IDataCursor inputCursor = input.getCursor(); IDataUtil.put(inputCursor, "input", " test test "); inputCursor.destroy(); Service.doInvoke("test.work", "Test_Flow", input);
<dependency> <groupId>commons-httpclient</groupId> <artifactId>commons-httpclient</artifactId> <version>3.1</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.5</version> </dependency>
package albert.practice.httpClient; import java.io.IOException; import org.apache.commons.httpclient.Credentials; import org.apache.commons.httpclient.HttpClient; import org.apache.commons.httpclient.HttpException; import org.apache.commons.httpclient.HttpStatus; import org.apache.commons.httpclient.UsernamePasswordCredentials; import org.apache.commons.httpclient.auth.AuthScope; import org.apache.commons.httpclient.methods.GetMethod; import org.apache.commons.httpclient.methods.PostMethod; import org.apache.commons.io.IOUtils; import com.google.common.base.Charsets; public class HttpClientExample { private String post_uri = "http://10.12.14.28:5555/rest/test.restful.RestTest2"; private String get_uri = "http://10.12.14.28:5555/rest/test.restful.RestTest3"; private String inputJson = "{\"ip\":\"10.10.12.66\",\"state\": \"OFFLINE\"}"; /** * Implements the HTTP POST method. * * @return response code * @throws HttpException * @throws IOException */ public int sendPost() throws HttpException, IOException { AuthScope authScope = getAuthScope(); Credentials credentials = getCredentials(); HttpClient client = new HttpClient(); client.getState().setCredentials(authScope, credentials); PostMethod postMethod = new PostMethod(post_uri); postMethod.setDoAuthentication(true); postMethod.setParameter("data", inputJson); return client.executeMethod(postMethod); } /** * Implements the HTTP GET method. * * @return the response string of the HTTP method * @throws HttpException * @throws IOException */ public String sendGet() throws HttpException, IOException { String responseString = ""; AuthScope authScope = getAuthScope(); Credentials credentials = getCredentials(); HttpClient client = new HttpClient(); client.getState().setCredentials(authScope, credentials); GetMethod getMethod = new GetMethod(get_uri + "?input=albert"); getMethod.setDoAuthentication(true); int statusCode = client.executeMethod(getMethod); if (HttpStatus.SC_OK == statusCode) { responseString = IOUtils.toString(getMethod.getResponseBodyAsStream(), Charsets.UTF_8); } return responseString; } /** * Set authentication scope. * * @return authentication scope */ private AuthScope getAuthScope() { String ip = "10.12.14.28"; int port = 5555; String realm = AuthScope.ANY_REALM; AuthScope authScope = new AuthScope(ip, port, realm); return authScope; } /** * Set the authentication credentials for the given scope. * * @return credentials */ private Credentials getCredentials() { String username = "cctv"; String password = "cctv"; Credentials credentials = new UsernamePasswordCredentials(username, password); return credentials; } }
package albert.practice.httpClient; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertNotNull; import java.io.IOException; import org.apache.commons.httpclient.HttpException; import org.apache.commons.httpclient.HttpStatus; import org.junit.Before; import org.junit.Test; public class HttpClientExampleTest { private HttpClientExample httpClientExample; @Before public void setup() { httpClientExample = new HttpClientExample(); } @Test public void testSendPost() throws HttpException, IOException { int statusCode = httpClientExample.sendPost(); assertEquals(HttpStatus.SC_OK, statusCode); } @Test public void testSendGet() throws HttpException, IOException { String responseString = httpClientExample.sendGet(); System.out.println("responseString = " + responseString); assertNotNull(responseString); } }
http://server:port/rest/FullyQualifiedResource
http://username:password@server:port/rest/FullyQualifiedResource
{"users":[{"name":"Albert","id":1},{"name":"Mandy","id":2}]}
1. Create a User object which have two attributes (id and name)
2. Create a getUsers method to retrieve dummy user collection
3. Create buildUserJson() to build JSON string from List of User
package albert.practice.json; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import org.json.JSONArray; import org.json.JSONObject; import lombok.AllArgsConstructor; import lombok.Data; import lombok.ToString; import lombok.extern.slf4j.Slf4j; @Slf4j public class JsonObjectTest { public static void main(String[] args) { JsonObjectTest test = new JsonObjectTest(); String userJSON = test.buildUserJson(); log.debug("user JSON = " + userJSON); } public String buildUserJson() { List<User> users = getUsers(); JSONObject dataset = new JSONObject(); users.stream().forEach(u -> addToDataset(u, dataset)); return dataset.toString(); } private static void addToDataset(User user, JSONObject dataset) { JSONObject userObj = new JSONObject(); userObj.put("id", user.getId()); userObj.put("name", user.getName()); // use the accumulate function to add to an existing value. The value // will now be converted to a list dataset.accumulate("users", userObj); } public List<User> getUsers() { User albert = new User(1, "Albert"); User mandy = new User(2, "Mandy"); return Arrays.asList(albert, mandy); } @Data @AllArgsConstructor @ToString private static class User { private Integer id; private String name; } }
public List<User> parseUserJson(String json) { JSONObject jsonObj = new JSONObject(json); JSONArray userArray = (JSONArray) jsonObj.get("users"); List<User> users = new ArrayList<>(); userArray.forEach(u -> addToList(u, users)); return users; } private void addToList(Object user, List<User> users) { JSONObject userObj = (JSONObject) user; Integer id = (Integer) userObj.get("id"); String name = (String) userObj.get("name"); users.add(new User(id, name)); }