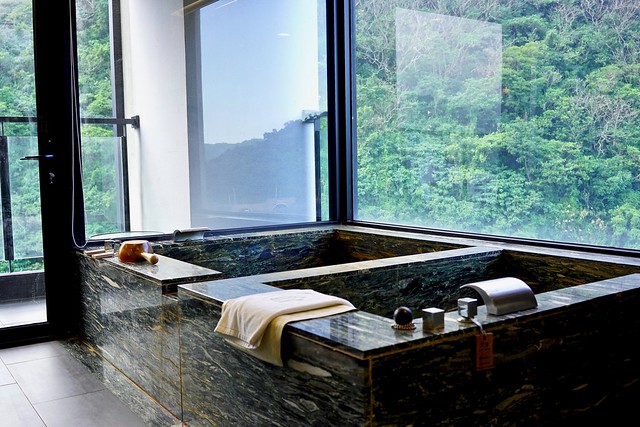
內埤海灣

豆腐岬風景區

粉鳥林


蜊埤湖


<html> <body> <p>Hello Albert! You have the following messages: <li><b>Tim:</b> Please don't forget to bring the laptop!</li> <li><b>Cindy:</b> Can you give me a visit this afternoon?</li> <li><b>Richard:</b> Don't forget the papers this time!</li> <li><b>Mom:</b> Don't forget the milk this time!</li> </p> </body> </html>
<html> <body> <p>Hello ${name}! You have the following messages: <#if messages??> <#-- if message has data, get data via iteration https://freemarker.apache.org/docs/ref_directive_if.html --> <#list messages as m> <li><b>${m.from}:</b> ${m.body}</li> </#list> <#else> <#-- if message is null, then show NO Message Found --> No Message Found! </#if> </p> </body> </html>
package com.test.batch; import com.test.batch.exception.FtlException; import freemarker.template.Configuration; import freemarker.template.Template; import freemarker.template.TemplateException; import lombok.Builder; import lombok.Getter; import lombok.extern.slf4j.Slf4j; import org.junit.Before; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.io.Writer; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; @RunWith(SpringRunner.class) @SpringBootTest @Slf4j public class FtlTest { private Configuration cfg; @Before public void init() { cfg = new Configuration(); // 設定到 classpath 讀取 ftl file cfg.setClassForTemplateLoading(this.getClass(), "/"); } @Test public void testMessageHtml() { try (Writer file = new FileWriter(new File("C:/ftl_message.html"));) { Template template = cfg.getTemplate("ftl/message.ftl"); Map<String, Object> data = new HashMap<>(); data.put("name", "Albert"); List<Message> messages = new ArrayList<>(); messages.add(Message.builder().from("Tim").body("Please don't forget to bring the laptop!").build()); messages.add(Message.builder().from("Cindy").body("Can you give me a visit this afternoon?").build()); messages.add(Message.builder().from("Richard").body("Don't forget the papers this time!").build()); messages.add(Message.builder().from("Mom").body("Don't forget the milk this time!").build()); data.put("messages", messages); template.process(data, file); } catch (IOException | TemplateException e) { throw new FtlException("fail to generate file from ftl file : " + e.getMessage(), e); } } @Getter @Builder public static class Message { private String from; private String body; } }
>java -jar batch-0.0.1-SNAPSHOT.jar -name Job1
package com.test.batch.job; import com.test.batch.service.Service1; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Slf4j @Component ("Job1") public class Job1 implements Job{ @Autowired private Service1 service1; public void execute() { log.debug("execute job1"); service1.sayHello(); } }
package com.test.batch.job; import lombok.extern.slf4j.Slf4j; import org.springframework.stereotype.Component; @Component("Job2") @Slf4j public class Job2 implements Job { @Override public void execute() { log.debug("execute job2"); } }
package com.test.batch.service; import com.test.batch.job.Job; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.ApplicationContext; import org.springframework.stereotype.Service; @Slf4j @Service public class JobExecutor { @Autowired private ApplicationContext ctx; public void execute(String argument) { try { // get bean by name Job job = (Job) ctx.getBean(argument); job.execute(); } catch (Exception e) { String error = "Fail to execute batch job, you provide job name is " + argument; log.error(error, e); } } }
package com.test.batch; import com.beust.jcommander.JCommander; import com.test.batch.parameter.BatchJobArgument; import com.test.batch.service.JobExecutor; import com.google.common.base.Strings; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.stereotype.Component; @Slf4j @Component public class BatchCommandLineRunner implements CommandLineRunner { @Autowired private JobExecutor jobExecutor; @Override public void run(String... args) throws Exception { BatchJobArgument arguments = new BatchJobArgument(); JCommander jCommander = JCommander.newBuilder().build(); jCommander.addObject(arguments); jCommander.parse(args); if(arguments.isHelp()){ jCommander.usage(); return; } log.debug("arguments = {}", arguments); if(Strings.isNullOrEmpty(arguments.getName())) { log.debug("Please assign job name (ex. -name Job1)"); }else{ jobExecutor.execute(arguments.getName()); } } }
package com.test.batch; import org.junit.jupiter.api.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.context.ApplicationContext; import org.springframework.test.context.junit4.SpringRunner; @RunWith(SpringRunner.class) @SpringBootTest class BatchApplicationTests { @Autowired private ApplicationContext ctx; @Test public void testCommandline() throws Exception { CommandLineRunner runner = ctx.getBean(CommandLineRunner.class); runner.run("-name", "Job2"); } }
CREATE OR REPLACE PACKAGE PKG_TEST AS /* TODO enter package declarations (types, exceptions, methods etc) here */ END PKG_TEST;
var o_message varchar2; var o_return_code number; exec package1.test_hello('Albert',:o_message, :o_return_code); print o_message; print o_return_code;
F:\git\batch-job\target>java -jar batch-0.0.1-SNAPSHOT.jar -name Job2
<properties> <java.version>1.8</java.version> <com.beust.jcommander.version>1.78</com.beust.jcommander.version> </properties> <dependencies> <dependency> <groupId>com.beust</groupId> <artifactId>jcommander</artifactId> <version>${com.beust.jcommander.version}</version> </dependency> </dependencies>
package com.test.batch.parameter; import com.beust.jcommander.Parameter; import lombok.Data; @Data public class BatchJobArgument { @Parameter(names = "-name", description = "batch job name") private String name; @Parameter(names = {"-help", "-h"}, help = true, description = "display help message") private boolean help; }
package com.test.batch; import com.beust.jcommander.JCommander; import com.test.batch.parameter.BatchJobArgument; import com.test.batch.service.JobExecutor; import com.google.common.base.Strings; import lombok.extern.slf4j.Slf4j; import org.springframework.boot.Banner; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @Slf4j @SpringBootApplication public class BatchApplication implements CommandLineRunner { private final JobExecutor jobExecutor; public BatchApplication(JobExecutor jobExecutor) { this.jobExecutor = jobExecutor; } public static void main(String[] args) { SpringApplication app = new SpringApplication(BatchApplication.class); app.setBannerMode(Banner.Mode.OFF); app.run(args); } @Override public void run(String... args) { BatchJobArgument arguments = new BatchJobArgument(); JCommander jCommander = JCommander.newBuilder().build(); jCommander.addObject(arguments); jCommander.parse(args); if(arguments.isHelp()){ jCommander.usage(); return; } log.debug("arguments = {}", arguments); if(Strings.isNullOrEmpty(arguments.getName())) { log.debug("Please assign job name (ex. -name Job1)"); }else{ jobExecutor.execute(arguments.getName()); } } }
[ main] com.test.batch.BatchApplication : arguments = BatchJobArgument(name=Job2)
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.0.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> </dependencies>Then using Spring's @SpringBootApplication annotation on our main class to enable auto-configuration.
package com.test.batch; import lombok.extern.slf4j.Slf4j; import org.springframework.boot.Banner; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @Slf4j @SpringBootApplication public class BatchApplication implements CommandLineRunner { private final JobExecutor jobExecutor; public BatchApplication(JobExecutor jobExecutor) { this.jobExecutor = jobExecutor; } public static void main(String[] args) { SpringApplication app = new SpringApplication(BatchApplication.class); app.setBannerMode(Banner.Mode.OFF); app.run(args); } @Override public void run(String... args) { // receive arguments in run method if (args != null && args.length > 0) { String argument = args[0]; log.debug("argument = {}", argument); jobExecutor.execute(argument); } else { log.debug("no argument found"); } } }
package com.esb.batch; import lombok.extern.slf4j.Slf4j; import org.springframework.boot.Banner; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @Slf4j @SpringBootApplication public class BatchApplication implements CommandLineRunner { public static void main(String[] args) { SpringApplication.run(BatchApplication.class, args); } }
package com.esb.batch; import lombok.extern.slf4j.Slf4j; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @Slf4j @SpringBootApplication public class BatchApplication implements CommandLineRunner { public static void main(String[] args) { SpringApplication app = new SpringApplication(BatchApplication.class); app.setBannerMode(Banner.Mode.OFF); app.run(args); } }